In Flutter, a button is a widget that allows the user to interact with the app by tapping on it. A button typically displays a text label or an icon, and it can be customized with various features such as colours, shapes, and actions.
Buttons in Flutter are built using the TextButton
, ElevatedButton
, or OutlineButton
classes, which are all subclasses of the Button
class. You can use these classes to create buttons with different styles and behaviour.
For example, you can use a TextButton
to create a simple button with no background colour or border, or a RaisedButton to create a button with a solid colour background and a shadow. You can also use an IconButton
to create a button with an icon, or a FloatingActionButton
to create a circular button that floats above the content of the app.
To use a button in Flutter, you can define it in the build
method of your widget and specify its properties such as the text label, icon, and onPressed callback. Here is an example of how to create a simple TextButton
:
TextButton(
child: Text('Button'),
onPressed: () {
// Perform action when button is pressed
},
)
There are a few types of buttons in Flutter, and every button can be customized like everything else in Flutter. I will add a few examples below of how to use the button.

Flutter TextButton
The base use of the TextButton in Flutter
In Flutter, the TextButton
is a button that displays a short text label and triggers an action when pressed. It is a simple and lightweight alternative to the FlatButton
and is often used in dialogs and forms.
From inside curly brackets {} in onPressed you call your custom function or do whatever you need.
Here’s an example of how you can use the TextButton
in a Flutter app:
TextButton(
style: TextButton.styleFrom(
textStyle: const TextStyle(fontSize: 20),
),
onPressed: () {
print('Button pressed!');
},
child: const Text('Enabled'),
),
This will create a button with the text “Enabled” that, when pressed, will print a message to the console. You can customize the appearance of the button by setting its style
and textTheme
properties.
You can also specify an autofocus
value to set whether the button should be focused when the app starts. This can be useful for accessibility purposes.
For more information on the TextButton
and other buttons available in Flutter, you can check out the official documentation:
https://api.flutter.dev/flutter/material/TextButton-class.html
Use the TextButton.icon in Flutter
TextButton.icon
is a widget in the Flutter framework that displays a button with an icon and a label. It is a subclass of the TextButton
class, which is a widget that displays a button with text.
The TextButton.icon
widget is useful when you want to display a button with both an icon and a text label. It allows you to specify the icon to display, as well as the text label, and provides an onPressed
callback that is called when the button is tapped.
Here’s an example of how to use a TextButton.icon
widget in a Flutter app:
TextButton.icon(
icon: Icon(Icons.add),
label: Text('Add'),
onPressed: () {
// Perform some action
},
)
This will display a button with an add icon and the text “Add”. When the button is tapped, the onPressed
callback will be called.
How to disable TextButton in Flutter
To disable a TextButton
in Flutter, you can set the onPressed
property to null
. This will prevent the button from triggering any action when it is pressed.
Here’s an example of how you can disable a TextButton
:
TextButton(
style: TextButton.styleFrom(
textStyle: const TextStyle(fontSize: 20),
),
onPressed: null,
child: const Text('Disabled'),
),
You can also use a boolean variable to enable or disable the button based on certain conditions. For example:
bool buttonEnabled = true;
TextButton(
onPressed: buttonEnabled ? () { print('Button pressed!'); } : null,
child: Text('Button'),
)
In this case, the button will be enabled when buttonEnabled
is true
, and disabled when buttonEnabled
is false
.
For more information on the TextButton
and other buttons available in Flutter, you can check out the official documentation:
https://api.flutter.dev/flutter/material/TextButton-class.html
Flutter TextButton with a gradient background and the border line
You can read more about a colour gradient in this article: How do you add a gradient background color in Flutter?
Stack(
children: <Widget>[
Positioned.fill(
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black, width: 1.0,),
gradient: LinearGradient(
begin: Alignment.topRight,
end: Alignment.bottomLeft,
colors: <Color>[
Color(0xFF0DA182),
Color(0xFF0A715C),
Color(0xFF12B876),
],
),
),
),
),
TextButton(
style: TextButton.styleFrom(
padding: const EdgeInsets.all(16.0),
foregroundColor: Colors.white,
textStyle: const TextStyle(fontSize: 20),
),
onPressed: () {},
child: const Text('Gradient'),
),
],
),
Flutter ElevatedButton with rounded border
ElevatedButton is a material design button that has an elevation and a raised button style in the Flutter framework. It is often used to represent a button that performs an action or initiates a dialogue. ElevatedButton can be customized using various properties, such as the colour and text of the button, the shape and size of the button, and the behaviour of the button when it is pressed or released.
Here is an example of how to use ElevatedButton in a Flutter app:
ElevatedButton(
onPressed: () {
// Perform some action when the button is pressed
},
child: Text('Press me'),
)

In this example, I used button elevation, shadow, border, text style, padding, border radius.
ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.red, // background
foregroundColor: Colors.white, // foreground
shadowColor: Colors.blue,
elevation: 10,
side: BorderSide(color: Colors.blue, width: 3),
shape: const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(30))),
textStyle: TextStyle(
color: Colors.white,
fontSize: 30,
fontWeight: FontWeight.w800,
fontStyle: FontStyle.italic
),
),
onPressed: () { },
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Text('Elevated Button'),
),
),
How to use FloatingActionButton in Flutter
In Flutter, a FloatingActionButton
is a button displayed in a circular shape that is typically anchored to the bottom right corner of the screen. It is typically used to perform a primary action in the app, such as creating a new item or sending a message.
This button usually is placed directly inside Scaffold as floatingActionButton.
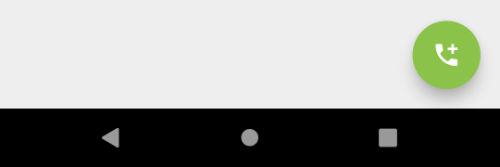
FloatingActionButton regular size (56×56)
By default, a FloatingActionButton
in Flutter has a size of 56×56 pixels. This size is defined by the mini
property, which is set to false
by default. If you want to create a smaller FloatingActionButton
, you can set the mini
property to true
. This will reduce the size of the button to 40×40 pixels.
floatingActionButton: FloatingActionButton(
mini: false,
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
FloatingActionButton small(40×40) or mini = true
floatingActionButton: FloatingActionButton.small(
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
//mini = true;
floatingActionButton: FloatingActionButton(
mini: true,
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
FloatingActionButton large(96×96)
floatingActionButton: FloatingActionButton.large(
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
Here you have a slightly modified FloatingActionButton
floatingActionButton: SizedBox(
height: 100.0,
width: 100.0,
child: FittedBox(
child: FloatingActionButton(
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
),
),
FloatingActionButton custom positions
Here you have predefined floatingActionButton positions
- floatingActionButtonLocation: FloatingActionButtonLocation.startDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.centerTop,
- floatingActionButtonLocation: FloatingActionButtonLocation.endDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.endFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.endTop,
- floatingActionButtonLocation: FloatingActionButtonLocation.startFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.startTop,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterTop,
- loatingActionButtonLocation: FloatingActionButtonLocation.miniEndDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniEndFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniEndTop,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniStartDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniStartFloat
- floatingActionButtonLocation: FloatingActionButtonLocation.miniStartTop
// floatingActionButtonLocation: FloatingActionButtonLocation.startDocked,
// floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
// floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat,
// floatingActionButtonLocation: FloatingActionButtonLocation.centerTop,
// floatingActionButtonLocation: FloatingActionButtonLocation.endDocked,
// floatingActionButtonLocation: FloatingActionButtonLocation.endFloat,
// floatingActionButtonLocation: FloatingActionButtonLocation.endTop,
// floatingActionButtonLocation: FloatingActionButtonLocation.startFloat,
// floatingActionButtonLocation: FloatingActionButtonLocation.startTop,
// floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterDocked,
// floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterFloat,
// floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterTop,
// floatingActionButtonLocation: FloatingActionButtonLocation.miniEndDocked,
// floatingActionButtonLocation: FloatingActionButtonLocation.miniEndFloat,
// floatingActionButtonLocation: FloatingActionButtonLocation.miniEndTop,
// floatingActionButtonLocation: FloatingActionButtonLocation.miniStartDocked,
floatingActionButtonLocation: FloatingActionButtonLocation.miniStartFloat,
// floatingActionButtonLocation: FloatingActionButtonLocation.miniStartTop,
floatingActionButton: FloatingActionButton(
elevation: 10,
backgroundColor: Colors.lightGreen,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
How to use IconButton in Flutter
An IconButton
is a button that displays an icon and responds to a tap gesture. It is a simple way to include a button with an icon in your Flutter app.
Here’s an example of how to use an IconButton
in a Flutter app:
IconButton(
icon: Icon(Icons.favorite),
onPressed: () {
// Do something when the button is pressed
},
)
In this example, the IconButton
displays a heart icon (Icons.favorite
) and calls a function when it is pressed. You can customize the appearance of the IconButton
by setting its color
, iconSize
, and other properties.
You can also specify the icon
property using a custom Widget
, such as a Text
widget or a custom Icon
widget. For example:
IconButton(
icon: Text('Press me'),
onPressed: () {
// Do something when the button is pressed
},
)
IconButton is used most of the time in Actions inside AppBar.
IconButton(
icon: const Icon(Icons.edit),
color: Colors.red,
onPressed: () {},
),
Instead of IconButton, you can use FloatedActionButton. It will work file.
FloatingActionButton(
child: Icon(
Icons.flip_camera_ios_outlined,
color: Colors.white,
),
onPressed: () {
},
)
How to use OutlineButton in Flutter
In Flutter, an OutlinedButton
is a button that displays a text label and an outline border around it. It displays a flat button with no background or elevation.
To use an OutlinedButton
in Flutter, you can simply create a new OutlineButton
widget and pass it the desired onPressed
callback and child
widget. Here’s an example:
OutlinedButton(
onPressed: () {
// Perform some action
},
child: Text('Button'),
)
This will create an OutlineButton
with the default style, which includes a text label with the default font style and an outline border.
How to use rounded OutlineButton in Flutter
To create a rounded outline button in Flutter, you can use the OutlinedButton
widget and set the shape
property to a RoundedRectangleBorder
with a desired borderRadius
.
Here is an example of how you can create a rounded outline button in Flutter:
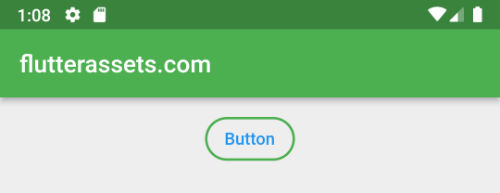
OutlinedButton(
style: OutlinedButton.styleFrom(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(18.0),
),
side: BorderSide(width: 2, color: Colors.green),
),
onPressed: () {},
child: Text('Button'),
)

OutlinedButton(
onPressed: () {},
style: ButtonStyle(
side: MaterialStateProperty.all(BorderSide(
color: Colors.red,
width: 3.0,
style: BorderStyle.solid)),
shape: MaterialStateProperty.resolveWith<OutlinedBorder?>((states) {
// Rounded button (when the button is pressed)
if (states.contains(MaterialState.pressed)) {
return RoundedRectangleBorder(borderRadius: BorderRadius.circular(15));
}
}
),
),
child: Text('OutlinedButton'),
),
What is the PopupMenuButton in Flutter
PopupMenuButton
is a widget in Flutter that displays a button with a three-dot icon, and when pressed, shows a menu with a list of options. It’s often used to provide additional options for an app in a compact and convenient way.
The PopupMenuButton
widget is a non-visual element that functions as a button, and when pressed, displays a menu with a list of options. You can customize the options that are displayed in the menu by setting the itemBuilder
property to a function that returns a list of PopupMenuItem
widgets. Each PopupMenuItem
should have a value
property and a child
property, where the value
is the value that will be returned when the menu item is selected, and the child
is the widget that will be displayed for the menu item.
When a menu item is selected, the PopupMenuButton
widget will call the onSelected
callback function, passing in the value
of the selected menu item as an argument. This allows you to handle the selection in your code and take any necessary actions.
Here’s an example of how to use a PopupMenuButton
:
PopupMenuButton<String>(
onSelected: (value) {
// handle menu item selection
},
itemBuilder: (BuildContext context) => <PopupMenuItem<String>>[
PopupMenuItem(
value: 'Option 1',
child: Text('Option 1'),
),
PopupMenuItem(
value: 'Option 2',
child: Text('Option 2'),
),
PopupMenuItem(
value: 'Option 3',
child: Text('Option 3'),
),
],
)
This code will display a button with a three-dot icon, and when pressed, will show a menu with the options “Option 1,” “Option 2,” and “Option 3.” When one of these options is selected, the onSelected
callback function will be called with the value of the selected menu item.
How to use PopupMenuButton in Flutter
I also mentioned PopupMenuButton in my article about AppBar which you can find here. I will add the same code here.

appBar: AppBar(
centerTitle: true,
title: Text("App Dropdown Menu"),
actions: [
//list if widget in appbar actions
PopupMenuButton(
icon: Icon(Icons.menu), //don't specify icon if you want 3 dot menu
color: Colors.blue,
itemBuilder: (context) => [
PopupMenuItem<int>(
value: 0,
child: Text("Setting",style: TextStyle(color: Colors.white),),
),
PopupMenuItem<int>(
value: 1, child: Text("Privacy Policy"),
),
PopupMenuItem<int>(
value: 2,
child: Row(
children: [
Icon(
Icons.logout,
color: Colors.yellow,
),
const SizedBox(
width: 7,
),
Text("Logout")
],
)),
],
onSelected: (item) => {print(item)},
),
],
),
//outside of build
void SelectedItem(BuildContext context, item) {
switch (item) {
case 0:
print("Settings");
// Navigator.of(context)
// .push(MaterialPageRoute(builder: (context) => SettingPage()));
break;
case 1:
print("Privacy Clicked");
break;
case 2:
print("User Logged out");
// Navigator.of(context).pushAndRemoveUntil(
// MaterialPageRoute(builder: (context) => LoginPage()),
// (route) => false);
break;
}
}
PopupMenuButton as floatingActionButton in Flutter
This code will display a floating action button with a three-dot icon, and when the button is pressed, a menu will appear with the options “Home,” “Settings,” and “About.” When one of these options is selected, the text in the center of the screen will change to reflect the selected menu item.
String _selectedMenuItem = 'Home';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Popup Menu Button Example'),
),
body: Center(
child: Text(_selectedMenuItem),
),
floatingActionButton: PopupMenuButton<String>(
onSelected: (value) {
setState(() {
_selectedMenuItem = value;
});
},
itemBuilder: (BuildContext context) => <PopupMenuItem<String>>[
PopupMenuItem(
value: 'Home',
child: Text('Home'),
),
PopupMenuItem(
value: 'Settings',
child: Text('Settings'),
),
PopupMenuItem(
value: 'About',
child: Text('About'),
),
],
),
);
}
How to use DropdownButton in Flutter
DropdownButton
is a widget in Flutter that displays a button with a drop-down arrow icon, and when pressed, shows a menu with a list of options. It’s often used to provide a list of selectable options in a compact and convenient way.
The DropdownButton
widget is a visual element that functions as a button, and when pressed, displays a menu with a list of options. You can customize the options that are displayed in the menu by setting the items
property to a list of DropdownMenuItem
widgets. Each DropdownMenuItem
should have a value
property and a child
property, where the value
is the value that will be returned when the menu item is selected, and the child
is the widget that will be displayed for the menu item.
When a menu item is selected, the DropdownButton
widget will call the onChanged
callback function, passing in the value
of the selected menu item as an argument. This allows you to handle the selection in your code and take any necessary actions.
Here’s an example of how to use a DropdownButton
:
String _selectedOption = 'Option 1';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
DropdownButton<String>(
value: _selectedOption,
onChanged: (value) {
setState(() {
_selectedOption = value!;
});
},
items: [
DropdownMenuItem(
value: 'Option 1',
child: Text('Option 1'),
),
DropdownMenuItem(
value: 'Option 2',
child: Text('Option 2'),
),
DropdownMenuItem(
value: 'Option 3',
child: Text('Option 3'),
),
],
)
],
),
);
}
This code will display a button with a drop-down arrow icon, and when pressed, will show a menu with the options “Option 1,” “Option 2,” and “Option 3.” When one of these options is selected, the onChanged
callback function will be called with the value of the selected menu item, and the button’s label will update to reflect the selected option.

Here is another example. First, initialize a list of items you will use.
List<DropdownMenuItem<String>> get dropdownItems{
List<DropdownMenuItem<String>> menuItems = [
DropdownMenuItem(child: Text("Please Select"),value: "0"),
DropdownMenuItem(child: Text("Apple"),value: "Apple"),
DropdownMenuItem(child: Text("Pear"),value: "Pear"),
DropdownMenuItem(child: Text("Orange"),value: "Orange"),
DropdownMenuItem(child: Text("Plum"),value: "Plum"),
];
return menuItems;
}
String selectedValue = "0";
Add your DropdownButton into your code.
DropdownButton(
value: selectedValue,
dropdownColor: Colors.orangeAccent,
icon: Icon(Icons.arrow_circle_down),
onChanged: (String? newValue){
setState(() {
selectedValue = newValue!;
});
},
items: dropdownItems
),
How to use InkWell as a button in Flutter
InkWell is a widget in Flutter that’s used to add touch feedback to a widget, typically a button. It adds a gesture recognizer and triggers an action when tapped.
When a user taps an InkWell
widget, it displays a splash effect that’s centered at the touch point and expands to fill the entire widget. It also highlights the widget, which can be customized using the highlightColor
property.
In addition to the visual effects, InkWell
also provides other functionality, such as the ability to handle taps, long presses, and gestures, and to trigger a callback when these events occur.
Here’s an example of how you can use InkWell
in a Flutter app:
InkWell(
onTap: () {
// Perform some action when the button is tapped
},
child: Container(
width: 200,
height: 50,
child: Text('Button'),
),
)
This will create a button that displays a splash effect and highlights when tapped and triggers the onTap callback. You can customize the appearance and behaviour of the InkWell
by specifying various properties, such as splashColor
, highlightColor
, and borderRadius
.
InkWell(
onTap: () {
// Perform some action when the button is tapped
},
splashColor: Colors.red,
highlightColor: Colors.yellow,
borderRadius: BorderRadius.circular(10),
child: Container(
width: 200,
height: 50,
child: Text('Button'),
),
)
This will create a button with a red splash effect when tapped, a yellow highlight effect when pressed, and rounded corners with a radius of 10 pixels.
Here is another simple example:
Material(
color: Theme.of(context).primaryColor,
child: InkWell(
splashColor: Theme.of(context).primaryColorLight,
child: Container(
height: 100,
),
onTap: () {},
),
)
I hope this article helped someone 🙂