What is a scrollable element in Flutter?
Flutter scrollable element is a widget that allows the user to scroll through a list of items or a single child that doesn’t fit on the screen. These widgets provide a scrollbar that the user can interact with by dragging or swiping, allowing them to scroll through the list or child.
Scrollable elements in Flutter include the SingleChildScrollView
, ListView
, PageView
, ListView.builder
, ListView.separated
, and ListView.custom
widgets. These widgets can be scrolled vertically or horizontally depending on the scrollDirection
property that is set.
For example, if you have a large amount of text in a Text
widget that doesn’t fit on the screen, you can use SingleChildScrollView
to make it scrollable.
SingleChildScrollView(
child: Text("Your long text here"),
)
Or if you have a list of images in a Column
widget and you want to make the list scrollable, you can use ListView
.
ListView(
children: <Widget>[
Image.asset("image1.jpg"),
Image.asset("image2.jpg"),
Image.asset("image3.jpg"),
],
)
These widgets allow users to view content that is larger than the available screen space by providing a scrollbar that can be interacted with.
How to make scrollable elements in Flutter
In Flutter, there are several widgets that you can use to make elements scrollable. The most common widgets used for this purpose are SingleChildScrollView
, ListView
, PageView
, ListView.builder
, ListView.separated
, and ListView.custom
.
SingleChildScrollView
is used when you want to make a single child widget scrollable. For example, if you have a large amount of text in a Text
widget that doesn’t fit on the screen, you can use SingleChildScrollView
to make it scrollable.
SingleChildScrollView(
child: Text("Your long text here"),
)
ListView
is used when you have a list of items that you want to make scrollable. For example, if you have a list of images in a Column
widget and you want to make the list scrollable, you can use ListView
.
ListView(
children: <Widget>[
Image.asset("image1.jpg"),
Image.asset("image2.jpg"),
Image.asset("image3.jpg"),
],
)
PageView
is similar to ListView
, but it is used for horizontally scrolling pages. For example, if you have a list of pages and you want to make them scrollable horizontally, you can use PageView
.
PageView(
children: <Widget>[
Container(color: Colors.red),
Container(color: Colors.green),
Container(color: Colors.blue),
],
)
ListView.builder
is similar to ListView
, but it is used when you have a large number of items and you want to make them scrollable efficiently.
ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return items[index];
},
)
ListView.separated
is similar to ListView
, but it is used when you want to add separators between items.
ListView.custom
is used when you want to create a custom scrollable list view.
All these widgets can be set to scroll vertically or horizontally by setting the scrollDirection
property.
ListView(
scrollDirection: Axis.vertical,
children: <Widget>[
// your scrollable children here
],
)
In summary, you can use SingleChildScrollView
for a single child widget, ListView
for a list of items, PageView
for a list of pages, ListView.builder
for a large number of items and ListView.separated
for a list of items with separators, and ListView.custom
for a custom scrollable list view.
More about these elements is below.
How to use SingleChildScrollView in Flutter
Flutter SingleChildScrollView
is a widget that allows you to make a single child widget scrollable. This is useful when you have a child widget that is too large to fit on the screen and you want the user to be able to scroll through it.
Here’s an example of how to use SingleChildScrollView
to make a Text
widget scrollable:
SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Text("Your long text here", style: TextStyle(fontSize: 60),),
),

In this example, the SingleChildScrollView
widget takes a single child, in this case, the Text
widget. The Text
widget contains a string of text that is too long to fit on the screen, so the SingleChildScrollView
makes it scrollable.
You can also use SingleChildScrollView
to make other types of widgets scrollable, such as an image or a container. For example, if you have an image that is too large to fit on the screen, you can wrap it in a SingleChildScrollView
to make it scrollable:
SingleChildScrollView(
child: Image.asset("your_image.jpg"),
)
You can also specify the scroll direction by setting the scrollDirection
property of the SingleChildScrollView
SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Image.asset("your_image.jpg"),
)
You can also specify the physics of the scrollable by setting the physics
property of the SingleChildScrollView
SingleChildScrollView(
physics: BouncingScrollPhysics(),
child: Image.asset("your_image.jpg"),
)
In summary, SingleChildScrollView
is a widget that allows you to make a single child widget scrollable. You can use it to make a Text
widget, an Image
widget, or other types of widgets scrollable. You can also specify the scroll direction and physics of the scrollable.
How to make a scrollable long Text in Flutter
In order to make a scrollable long text in Flutter, you can use the SingleChildScrollView
widget along with the Text
widget. The SingleChildScrollView
widget allows you to create a scrollable area that can only contain a single child.
Here is an example of how to use the SingleChildScrollView
widget to create a scrollable long text:
SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Text(
'Your long text here... Lorem ipsum dolor sit amet, consectetur adipiscing elit.',
style: TextStyle(fontSize: 30),
),
)
The SingleChildScrollView
widget by default scrolls vertically, and to make it scroll horizontally, you need to set the scrollDirection
property to Axis.horizontal
.
By setting scrollDirection: Axis.horizontal
, the text will be scrollable horizontally if it exceeds the width of the screen or the parent container. Also, if you are facing an issue with scrolling, please check if the SingleChildScrollView
is wrapped inside a parent widget that has a defined width and height, as the scrolling only works when the SingleChildScrollView
is given a size.
What is the scrollDirection in SingleChildScrollView in Flutter
In Flutter, the scrollDirection
property of the SingleChildScrollView
widget is used to specify the direction in which the child widget can be scrolled. The scrollDirection
property takes an Axis
value, which can be either Axis.horizontal
or Axis.vertical
.
- If the
scrollDirection
is set toAxis.vertical
, the child widget can be scrolled vertically, meaning the user can scroll up and down through the child. This is the default value. - If the
scrollDirection
is set toAxis.horizontal
, the child widget can be scrolled horizontally, meaning the user can scroll left and right through the child.
Here’s an example of how to use the scrollDirection
property to make a Text
widget scrollable horizontally:
SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Text("Your long text here", style: TextStyle(fontSize: 60),),
)

In this example, the SingleChildScrollView
widget has its scrollDirection
property set to Axis.horizontal
, so the child Text
widget can be scrolled horizontally.
It’s worth noting that SingleChildScrollView
widget only allows scrolling in one direction at a time, so if you set the scrollDirection
to Axis.horizontal
the child can’t be scrolled vertically and vice versa.
How to make a scrollable row widget in Flutter
In order to make a scrollable row widget in Flutter that uses images as children, you can use the SingleChildScrollView
widget. The SingleChildScrollView
widget allows you to create a scrollable area that can only contain a single child.
Here is an example of how to use the SingleChildScrollView
widget to create a scrollable row of images:
SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Row(
children: <Widget>[
Image.network('https://picsum.photos/250?image=9'),
Image.network('https://picsum.photos/250?image=9'),
Image.network('https://picsum.photos/250?image=9'),
Image.network('https://picsum.photos/250?image=9'),
Image.network('https://picsum.photos/250?image=9'),
],
),
)
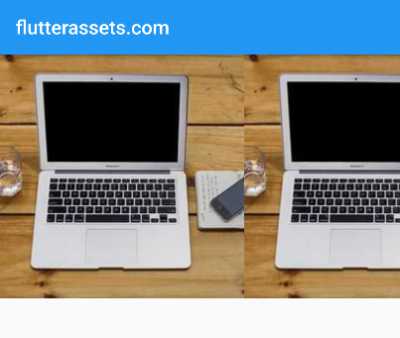
In this example, the SingleChildScrollView
widget takes a scrollDirection
property set to Axis.horizontal
, this makes the row of images scrollable horizontally. The child
property takes a Row
widget that contains a list of Image
widgets that are loaded from the network using the Image.network
constructor. You can replace the URLs of the images with your own URLs or assets, and also you can use a list of images instead of hard coding them. You can also adjust the width and height of the images to fit your design.
This will create a scrollable row of images that you can scroll horizontally. You can adjust the SingleChildScrollView
widget to suit your needs and design.
Scrollable elements with a ListView in Flutter
The ListView
widget in Flutter can be used to make a list of elements scrollable. The ListView
widget takes a list of children, and it makes them scrollable in the direction specified by the scrollDirection
property. The default value is Axis.vertical
, which means the list can be scrolled vertically.
Here’s an example of how to use the ListView
widget to make a list of images scrollable:
ListView(
children: <Widget>[
Image.asset("assets/logo.png"),
Image.asset("assets/logo.png"),
Image.asset("assets/logo.png"),
Image.asset("assets/logo.png"),
],
)

In this example, the ListView
widget takes a list of children, which are Image
widgets. The ListView
makes these images scrollable in the vertical direction.
You can also make the list scrollable horizontally by setting the scrollDirection
property to Axis.horizontal
:
ListView(
scrollDirection: Axis.horizontal,
children: <Widget>[
Image.asset("assets/logo.png"),
Image.asset("assets/logo.png"),
Image.asset("assets/logo.png"),
Image.asset("assets/logo.png"),
],
)
You can also use the ListView.builder
which allows you to create a scrollable list of items that are built on demand.
ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return items[index];
},
)
The ListView.builder
takes an itemCount
property that represents the number of items in the list, and an itemBuilder
callback that’s called for each item. This way, the items are built on demand as the user scrolls through the list. This can be more efficient when you have a large number of items.
In summary, ListView
is a widget that allows you to make a list of elements scrollable in Flutter. You can use it to create a vertically or horizontally scrollable list of elements, such as images or text. You can also use ListView.builder
for an efficient way of creating scrollable list views when you have a large number of items.
ListView.builder with images in Flutter
Here’s an example of how to use the ListView.builder
to create a scrollable list of images with 5 items:
ListView.builder(
itemCount: 5,
itemBuilder: (context, index) {
return Image.asset("image$index.jpg");
},
)
In this example, the ListView.builder
takes an itemCount
of 5 and an itemBuilder
callback function. The itemCount
property tells the ListView.builder
that there are 5 items in the list. The itemBuilder
function is called for each item in the list, and it returns an Image
widget with the asset name image$index.jpg
. The $index will be replaced by 0, 1, 2, 3 and 4 respectively. The images will be shown in the list one after another.
As the user scrolls through the list, the itemBuilder
function is called to build the next item. This can be more efficient than creating all the items upfront, especially when you have a large number of items.
In summary, ListView.builder
allows you to create a scrollable list view efficiently. In this example, it creates a scrollable list of 5 images, where the images are built on demand as the user scrolls through the list.
How to make a scrollable row with images in Flutter
In Flutter, you can use the ListView
widget to create a scrollable row of items, including images.
One way to create a scrollable row of images in Flutter is to use the ListView.builder
widget and pass a List
of image URLs or assets to the itemBuilder
callback function. The ListView.builder
widget takes an itemCount
property which is the number of items in the list, and an itemBuilder
callback function which is called for each item in the list.
Here’s an example of how to use the ListView.builder
widget to create a scrollable row of images:
Container(
height: 150,
child: ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: imageList.length,
itemBuilder: (context, index) {
return Container(
margin: EdgeInsets.all(5),
width: 150,
height: 150,
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(imageList[index]),
fit: BoxFit.cover,
),
),
);
},
),
)

In this example, the ListView.builder
widget takes an itemCount
property which is set to the length of the imageList
. The itemBuilder
callback function takes context
and index
and returns a Container
widget which has a width and height of 150, and a BoxDecoration
with an image
property which is set to the image from the imageList
at the index
position.
Here’s an example of how to create an image list for the example above:
final List<String> imageList = [
'https://picsum.photos/250?image=9',
'https://picsum.photos/250?image=9',
'https://picsum.photos/250?image=9',
'https://picsum.photos/250?image=9',
'https://picsum.photos/250?image=9',
'https://picsum.photos/250?image=9',
];
In this example, imageList
is a List
of String
s that contain URLs of images.
Scrollable elements with a ListView.separated in Flutter
In Flutter, the ListView.separated
widget can be used to create a scrollable list of items, where each item is separated by a separator. The separator can be any widget, such as a line, a divider, or any other widget.
Here’s an example of how to use the ListView.separated
widget to create a scrollable list of text items, separated by a divider:
ListView.separated(
itemCount: 5,
itemBuilder: (context, index) {
return Text("Item $index");
},
separatorBuilder: (context, index) {
return Divider();
},
)

In this example, the ListView.separated
widget takes an itemCount
of 5, an itemBuilder
callback function, and a separatorBuilder
callback function. The itemCount
property tells the ListView.separated
that there are 5 items in the list. The itemBuilder
function is called for each item in the list and it returns a Text
widget with the label “Item $index”. The $index will be replaced by 0, 1, 2, 3 and 4 respectively.
The separatorBuilder
function is called for each separator between items. It returns a Divider
widget which is used as a separator between the items.
As the user scrolls through the list, the itemBuilder
and separatorBuilder
functions are called to build the next item and separator. This can be more efficient than creating all the items upfront, especially when you have a large number of items.
You can also use ListView.separated
with other types of widgets and customize the separator as you want.
In summary, ListView.separated
is a widget that allows you to create a scrollable list of items with separators in Flutter. It works similarly to ListView.builder
, but it allows you to add a separator between each item, which can be any widget. The separator widget is built on demand as the user scrolls through the list, making it efficient when you have a large number of items.
ListView.separated with divider every fifth item in Flutter
Here’s an example of how to use the ListView.separated
widget to create a scrollable list of text items with a Container
as a divider after every fifth item:
ListView.separated(
itemCount: 20,
separatorBuilder: (context, index) {
if ((index + 1) % 5 == 0) {
return Container(
height: 50,
color: Colors.grey,
);
}
return SizedBox(height: 0);
},
itemBuilder: (context, index) {
return Text("Item $index");
},
)

In this example, the ListView.separated
widget takes an itemCount
property which is set to 20, an itemBuilder
and separatorBuilder
. The itemBuilder
is creating a Text
widget with “Item $index” as its text for each index passed to it. The separatorBuilder
is checking if the index is divisible by 5, if it is it returns a Container
widget with a height of 50 and a grey colour, otherwise it returns an empty SizedBox
widget with a height of 0.
You can customize the Container
by changing its properties, such as width, height, colour, etc.
It’s important to check the logic of your separatorBuilder function, in the example above the first item will not have a divider before it, so you may want to add a conditional statement to handle that case.
Flutter ListView.separated insert the ad in every nth row
Here’s an example of how to insert an ad in every 4th row in a ListView.separated with an AdWidget that has a container and text in it:
final n = 4;
ListView.separated(
itemCount: 20,
separatorBuilder: (context, index) => SizedBox(height: 0),
itemBuilder: (context, index) {
if (index % n == 0) {
return AdWidget();
} else {
return Text("Item $index");
}
},
)
In this example, the ListView.separated
widget takes an itemCount
property which is set to 20, an itemBuilder
and separatorBuilder
. The itemBuilder
is creating a Text
widget with “Item $index” as its text for each index passed to it and also checking if the current index is divisible by n
using the modulo operator(%), if it is, it returns an AdWidget()
, otherwise, it returns a Text
widget. The separatorBuilder simply returns an empty SizedBox
widget with a height of 0, this way we don’t need to use any container as a separator.
Here’s an example of how to create a simple AdWidget
with a Container
and Text
in it:
class AdWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
width: double.infinity,
height: 100,
color: Colors.yellow,
child: Center(
child: Text('Ad', style: TextStyle(fontSize: 22, color: Colors.black)),
),
);
}
}

In this example, the AdWidget
is a stateless widget that returns a Container
widget with a width and height of 100 and yellow colour. Inside the container, there is a Center widget that centres the child which is a Text widget with “Ad” as its text and black colour. You can customize the AdWidget to your needs, for example, you can add an image, a video, or a more complex UI.
It’s important to keep in mind that the itemCount
should be divisible by n
because the last element of the listview may be an ad, and it’s usually not desired.
Also, it’s important to check the logic of your itemBuilder function, in the example above the first item will not have an ad before it, so you may want to add a conditional statement to handle that case.
You can also use a while
loop to create a list of items that have a fixed number of items between each ad, this way you can have more control over the number of ads and items in the list.
Scrollable elements with a ListView.custom in Flutter
In Flutter, the ListView.custom
widget is used to create a scrollable list of items that use a custom scroll physics. This can be useful when you need to create a list of items that have a unique scrolling behaviour, such as a parallax scrolling effect.
ListView.custom
requires a SliverChildDelegate
to manage the children and a ScrollController
to control the scrolling. A SliverChildDelegate
is a delegate that controls the layout of children in a sliver.
Here’s an example of how to use the ListView.custom
widget to create a scrollable list of text items with a custom scroll physics:
ListView.custom(
controller: ScrollController(),
physics: MyCustomScrollPhysics(),
childrenDelegate: SliverChildBuilderDelegate(
(context, index) {
return Text("Item $index", style: TextStyle(fontSize: 30),);
},
childCount: 35,
),
)
In this example, the ListView.custom
widget takes a controller
and physics
. The controller
is a ScrollController
that controls the scrolling and the physics
is a MyCustomScrollPhysics
which is a custom scroll physics that you can define or use one of the provided ones like BouncingScrollPhysics
or ClampingScrollPhysics
.
The ListView.custom
widget also takes a childrenDelegate
, which is a SliverChildBuilderDelegate
that controls the layout of children in a
sliver. The SliverChildBuilderDelegate
in this example is creating a Text
widget with “Item $index” as its text for each index passed to it. The childCount
property of the SliverChildBuilderDelegate
is set to 5, so it will create 5 children in total.
It’s important to note that when using ListView.custom
the layout of the children is controlled by the childrenDelegate
and not by the ListView.custom
widget itself, this allows you to have more flexibility and control over the layout and the scrolling behaviour.
In this example, the ListView.custom
widget is using MyCustomScrollPhysics
as its physics, but you can also use one of the provided physics classes like BouncingScrollPhysics
, ClampingScrollPhysics
, FixedExtentScrollPhysics
, NeverScrollableScrollPhysics
, PageScrollPhysics
, etc.
It’s important to test the different physics and choose the one that suits best your use case and provides a good user experience.
Here is an example of a simple custom physics class:
class MyCustomScrollPhysics extends ScrollPhysics {
@override
double applyPhysicsToUserOffset(ScrollMetrics metrics, double offset) {
// Modify the offset here to create your custom scrolling behavior
return offset * 0.5;
}
}
Flutter scroll physics
In Flutter, there are several built-in scroll physics classes that you can use to control the scrolling behaviour of a ListView
or PageView
widget. These classes are found in the scroll_physics
package.
Here are some of the most commonly used scroll physics classes in Flutter:
BouncingScrollPhysics
: This physics class creates a bouncy overscroll effect when the user scrolls past the edge of the list. This is the default physics class for scrollable widgets.ClampingScrollPhysics
: This physics class stops the scroll at the edge of the list without overscrolling.FixedExtentScrollPhysics
: This physics class is used for fixed-extent items, such as items in a horizontalListView
where all items have the same width.NeverScrollableScrollPhysics
: This physics class disables scrolling for the list.PageScrollPhysics
: This physics class is used forPageView
widget, it’s suitable for horizontally scrolling pages.ScrollPhysics
: This is the base class for all scroll physics. It’s not directly used, but it provides some useful methods that you can override to create your own custom scroll physics.
It’s worth mentioning that the available physics classes are not mutually exclusive, you can combine some of them to achieve different effects. For example, you can use BouncingScrollPhysics
as the outer scroll physics and FixedExtentScrollPhysics
as the inner scroll physics.
Additionally, you can also create your own custom scroll physics class by extending ScrollPhysics
and overrides the methods to achieve the desired behavior. This requires a good understanding of the physics of scrolling and the Flutter framework, and it’s not recommended if you can achieve the desired behavior using one of the provided physics classes.
Scrollable PageView in Flutter
In Flutter, the PageView
widget is used to create a horizontally scrollable list of pages. Each page can contain any type of content, such as text, images, or a combination of both. The PageView
widget is similar to the ListView
widget, but it is specifically designed for scrolling pages horizontally.
Here’s an example of how to use the PageView
widget to create a horizontally scrollable list of pages:
PageView(
children: <Widget>[
Container(color: Colors.red),
Container(color: Colors.green),
Container(color: Colors.blue),
],
)
In this example, the PageView
widget takes a list of children, which are Container
widgets. The PageView
makes these containers as pages that can be scrolled horizontally. Each container has a different colour, so you can see the different pages.
You can also use PageView.builder
which allows you to create pages on demand.
PageView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return items[index];
},
)
The PageView.builder
takes an itemCount
property that represents the number of items(pages) in the list, and an itemBuilder
callback that’s called for each item. This way, the pages are built on demand as the user scrolls through the pages. This can be more efficient when you have a large number of pages.
You can also specify the physics of the scrollable by setting the physics
property of the PageView
PageView(
physics: BouncingScrollPhysics(),
children: <Widget>[
Container(color: Colors.red),
Container(color: Colors.green),
Container(color: Colors.blue),
],
)
In summary, PageView
is a widget that allows you to create a horizontally scrollable list of pages in Flutter. You can use it to create pages with any type of content, such as text, images, or a combination of both. You can also use PageView.builder
for an efficient way of creating scrollable page views when you have a large number of pages. Additionally, you can specify the physics of the scrollable.
PageView.builder with images in Flutter
Here’s an example of how to use the PageView.builder
to create a horizontally scrollable list of images with 5 items:
PageView.builder(
itemCount: 5,
itemBuilder: (context, index) {
return Image.asset("image$index.jpg");
},
)
In this example, the PageView.builder
takes an itemCount
of 5 and an itemBuilder
callback function. The itemCount
property tells the PageView.builder
that there are 5 pages(items) in the list. The itemBuilder
function is called for each page in the list, and it returns an Image
widget with the asset name image$index.jpg
. The $index will be replaced by 0, 1, 2, 3 and 4 respectively. The images will be shown as pages one after another.
As the user scrolls through the pages, the itemBuilder
function is called to build the next page. This can be more efficient than creating all the pages upfront, especially when you have a large number of pages.
In summary, PageView.builder
allows you to create a horizontally scrollable list of pages efficiently. In this example, it creates a scrollable list of 5 images as pages, where the images are built on demand as the user scrolls through the pages. PageView.builder
works like ListView.builder
but it’s specific for horizontally scrolling pages.