What is a hyperlink
A hyperlink, also known as a link, is a reference to a web page or other resource that the user can follow by clicking or tapping. Hyperlinks are typically displayed as underlined or coloured text, but they can also be represented by buttons, images, or other types of UI elements. When a user clicks or taps on a hyperlink, they are taken to the web page or resource that the link references.
Hyperlinks are an essential part of the web, as they allow users to easily navigate between different pages and resources. Hyperlinks can also be used to link to specific parts of a web page, called fragment links, and to files, such as pdfs, images, videos, etc.
Where the hyperlinks can be used in Flutter
Hyperlinks can be used in a variety of ways in a Flutter app, some examples include:
- Navigation: Hyperlinks can be used to navigate between different pages or screens in the app. For example, you can use a hyperlink in a Text widget to navigate to a specific page when tapped on.
- Web pages: Hyperlinks can be used to open web pages in the default browser. For example, you can use a hyperlink in a Text widget to open a website when tapped on.
- Email and phone: Hyperlinks can be used to open email and phone apps. For example, you can use a hyperlink in a Text widget to open the email app with a specific email address or open the phone app to call a specific number when tapped on.
- Social media: Hyperlinks can be used to open social media apps. For example, you can use a hyperlink in a Text widget to open a specific profile on social media when tapped on.
- App content: Hyperlinks can be used to open specific content within the app. For example, you can use a hyperlink in a Text widget to open a specific article or video within the app when tapped on.
- PDF or document: Hyperlinks can be used to open pdf or document within the app or in the default app.
These are just a few examples of how hyperlinks can be used in a Flutter app, the possibilities are endless.
Creating a Hyperlink Word in Text using Flutter
Flutter provides several widgets that can be used to create a text with a hyperlink or clickable word. One way to do this is by using the RichText
widget and the InkWell
widget.
The RichText
widget allows you to style different parts of the text differently. You can use the TextSpan
widget to specify the part of the text that you want to make clickable, and then use the gestureRecognizers
property to add a gesture recognizer that launches a URL when the text is tapped.
The InkWell
widget is a Material Design widget that detects taps and provides visual feedback. You can use the onTap
property to specify a callback function that will be called when the user taps on the clickable word.
You can also use the InkWell
widget with Text
and wrap the text with InkWell
to make it interactive, you can also use a Wrap
or Row
widget to separate the text and the clickable word.
To open the URL you can use the launchUrl
function from the url_launcher
package.
Text widget with a hyperlink in Flutter
In Flutter, you can create a Text widget with a hyperlink by using the InkWell
widget and the url_launcher
package. Here are the steps you can follow:
Import the necessary package. To use the url_launcher
package, you will need to add the following line at the top of your Dart file:
import 'package:url_launcher/url_launcher.dart';
Also do not forget to add the package to the pubspec.yaml file:
dependencies:
url_launcher: ^6.1.8
Create an InkWell
widget. The InkWell
widget is a Material Design widget that detects taps and provides visual feedback. You can use the onTap
property to specify a callback function that will be called when the user taps on the text.
InkWell(
onTap: _launchURL,
child: const Text(
'Click here to open a website',
style: TextStyle(
fontSize: 30,
color: Colors.blue,
decoration: TextDecoration.underline,
),
),
),
Define the callback function. This function will be called when the user taps on the text. You can use the launch
function from the url_launcher
package to open a URL in the default browser.
_launchURL() async {
Uri _url = Uri.parse('https://www.google.com');
if (await launchUrl(_url)) {
await launchUrl(_url);
} else {
throw 'Could not launch $_url';
}
}

Use the Text widget in your code. You can use the InkWell
widget with Text
in your code, as you would any other widget, and when the user taps on the text, it will open the specified URL in the default browser.
InkWell
is wrapped around the Text
widget, it detects the tap on the text and calls the _launchURL
function, this allows the text to have visual feedback when it’s tapped, and also to open the link. You can customize the widget further to suit your needs, like changing the text, text style, and the link.
How to create RichText with a hyperlink in Flutter
In Flutter, you can create a RichText widget with a hyperlink by using the RichText
widget, the TextSpan
widget and the url_launcher
package. Here are the steps you can follow.
Import the necessary package. To use the url_launcher
package, you will need to add the following line at the top of your Dart file:
import 'package:url_launcher/url_launcher.dart';
add the package to your pubspec.yaml file:
dependencies:
url_launcher: ^6.1.8
Create a RichText
widget that contains the text you want to display, including the clickable word. You can use the TextSpan
widget to specify the part of the text that you want to make clickable.
TextSpan(
children: [
TextSpan(text: 'This is a ', style: TextStyle(color: Colors.black)),
TextSpan(
text: 'clickable word',
style: TextStyle(
color: Colors.blue,
decoration: TextDecoration.underline,
),
),
TextSpan(text: '.'),
],
),
Add a gesture recognizer to the TextSpan
widget. A gesture recognizer is a widget that can recognize specific gestures on the screen and trigger a callback function when the gesture is detected. You can use the onTap
property of the gesture recognizer to specify the callback function that will be called when the user taps on the clickable word.
RichText(
text: TextSpan(
children: [
TextSpan(text: 'This is a ', style: TextStyle(color: Colors.black)),
TextSpan(
text: 'clickable word',
style: TextStyle(
color: Colors.blue,
decoration: TextDecoration.underline,
),
recognizer: TapGestureRecognizer()..onTap = _launchURL,
),
TextSpan(text: '.'),
],
),
)
Define the callback function that will be called when the user taps on the clickable word. This function can use the launchUrl
function from the url_launcher
package to open a URL in the default browser.
_launchURL() async {
Uri _url = Uri.parse('https://www.google.com');
if (await launchUrl(_url)) {
await launchUrl(_url);
} else {
throw 'Could not launch $_url';
}
}
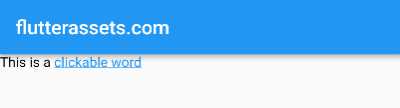
By following these steps you will create a RichText widget that contains a clickable word, once the user clicks on the word it will open the specified URL in the default browser.
It’s important to note that the TextSpan
widget is used to style a part of the text differently, and the gestureRecognizer
property allows you to associate a gesture recognizer with the TextSpan.
You can also customize the widget further to suit your needs, for example, you can change the text, text style, and the link. And you can also use this approach to make multiple words hyperlinks in one RichText.
It’s also important to mention that the RichText
widget is a paragraph of mixed-style text. It displays text that uses multiple different styles. The text to display is described using a tree of TextSpan
widgets, each of which has an associated style that is used for that subtree.
How to create a hyperlink text with the Wrap widget in Flutter
In Flutter, you can create a sentence with a hyperlink and one clickable word by using the Wrap
widget, the Text
widget, and the url_launcher
package. Here’s an example of how you can do this.
Import the necessary package. To use the url_launcher
package, you will need to add the following line at the top of your Dart file:
import 'package:url_launcher/url_launcher.dart';
And add it to the dependencies in your pubspec.yaml file:
dependencies:
url_launcher: ^6.1.8
Create a Wrap
widget that contains the text you want to display, including the clickable word. You can use Text
widgets to create the text and then use the InkWell
widget to make the clickable word interactive.
Wrap(
children: <Widget>[
Text('This is a '),
InkWell(
onTap: _launchURL,
child: Text('clickable word',
style: TextStyle(
color: Colors.blue,
decoration: TextDecoration.underline,
),
),
),
Text('.'),
],
)
Define the callback function that will be called when the user taps on the clickable word. This function can use the launch
function from the url_launcher
package to open a URL in the default browser.
_launchURL() async {
Uri _url = Uri.parse('https://www.google.com');
if (await launchUrl(_url)) {
await launchUrl(_url);
} else {
throw 'Could not launch $_url';
}
}
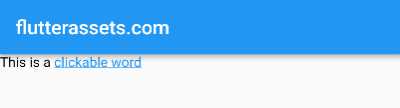
By following these steps, you will create a sentence with a clickable word. The Wrap
widget is used to contain the text and separates the text from the clickable word. the InkWell
widget is wrapped around the Text
widget of the clickable word, it detects the tap on the text and calls the _launchURL
function, which allows the text to have visual feedback when it’s tapped and also to open the link when clicked on.
You can customize the widget further to suit your needs, like changing the text, text style, and the link.
How to open an email in Flutter with a Hyperlink
In Flutter, you can open an email with a hyperlink by using the mailto
protocol and the url_launcher
package. Here’s an example of how you can do this.
Import the necessary package. To use the url_launcher
package, you will need to add the following line at the top of your Dart file:
import 'package:url_launcher/url_launcher.dart';
Add it to the dependencies in your pubspec.yaml file:
dependencies:
url_launcher: ^6.1.8
Create a InkWell
widget with a Text and wrap it around the email address. You can use the onTap
property to specify a callback function that will be called when the user taps on the email address.
InkWell(
onTap: _launchEmail,
child: Text(
'example@gmail.com',
style: TextStyle(
color: Colors.blue,
decoration: TextDecoration.underline,
),
),
),
Define the callback function that will be called when the user taps on the email address. This function can use the launch
function from the url_launcher
package to open the email app with the specified email address.
_launchEmail(){
final Uri emailLaunchUri = Uri(
scheme: 'mailto',
path: 'our.email@gmail.com',
queryParameters: {
'subject': 'Testing subject',
'body': 'Testing body text'
},
);
launchUrl(emailLaunchUri);
}
In this example, the _launchEmail
function constructs the mailto url using the email address, subject, and body provided and uses the launchUrl
function from the url_launcher
package to open the email app with the specified email address, subject and body. You can customize this function further to suit your needs, like adding cc, bcc or attachments.
It’s important to note that this will open the default email app on the device, if it’s not set it will show an error message.
How to Open Phone App with Hyperlink in Flutter
In Flutter, you can open the phone app with a hyperlink by using the tel
protocol and the url_launcher
package. Here’s an example of how you can do this.
Import the necessary package. To use the url_launcher
package, you will need to add the following line at the top of your Dart file:
import 'package:url_launcher/url_launcher.dart';
Add it to the dependencies in your pubspec.yaml file:
dependencies:
url_launcher: ^6.1.8
Create a InkWell
widget with a Text and wrap it around the phone number. You can use the onTap
property to specify a callback function that will be called when the user taps on the phone number.
InkWell(
onTap: _launchPhone,
child: Text(
'1234567890',
style: TextStyle(
color: Colors.blue,
decoration: TextDecoration.underline,
),
),
)
Define the callback function that will be called when the user taps on the phone number. This function can use the launch
function from the url_launcher
package to open the phone app with the specified phone number.
_launchPhone() async {
final phone = Uri.parse('tel:1234567890');
if (await canLaunchUrl(phone)) {
await launchUrl(phone);
} else {
throw 'Could not launch $phone';
}
}
In this example, the _launchPhone
function constructs the tel url using the phone number provided and uses the launch
function from the url_launcher
package to open the phone app with the specified phone number. You can customize this function further to suit your needs, like adding different phone numbers or phone numbers with different codes.
Important
To make the hyperlink that opens the phone app work on Android devices, you need to add some information to a file called AndroidManifest.xml.
Here are the steps:
- Go to the folder android/app/src/main/ in your project.
- Find the file AndroidManifest.xml and open it.
- Add the <queries> element as a child of the root element.
- Add the URL schemes you pass to the canLaunch function inside the <queries> element.
This step is important starting on Android 11 (API 30) or higher, otherwise, the hyperlink won’t work.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.url_launcher_example">
<queries>
<!-- To open phone's dialer app -->
<intent>
<action android:name="android.intent.action.DIAL" />
<data android:scheme="tel" />
</intent>
<!-- To send SMS messages -->
<intent>
<action android:name="android.intent.action.SENDTO" />
<data android:scheme="smsto" />
</intent>
<!-- To open email app -->
<intent>
<action android:name="android.intent.action.SEND" />
<data android:mimeType="*/*" />
</intent>
<!-- To opens https URLs -->
<intent>
<action android:name="android.intent.action.VIEW" />
<data android:scheme="https" />
</intent>
</queries>
<application
...
</application>
</manifest>