What is a Flutter ElevatedButton
In Flutter, an ElevatedButton
is a widget that represents a button with a raised effect, similar to a material design button. It’s part of the flutter:material
library and can be used to add a button with a raised effect to a user interface.
Here’s an example of how you can use an ElevatedButton
in a Flutter app:
ElevatedButton(
onPressed: () {
// Do something when the button is pressed
},
child: Text('Button'),
)
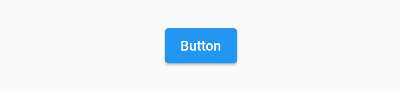
You can customize the appearance of the ElevatedButton
by using its various properties, such as style
, color
, and elevation
. For example:
ElevatedButton(
onPressed: () {
// Do something when the button is pressed
},
child: Text('Button'),
style: ElevatedButton.styleFrom(
backgroundColor: Colors.blue,
foregroundColor: Colors.white,
elevation: 5
),
)

This would create a button with white background, blue text, and an elevation of 5.
What is a raised effect in Flutter?
A raised effect in Flutter refers to the visual appearance of a button or other widget that looks like it is raised above the surface of the screen. This is often achieved by using a drop shadow or other graphical effect to give the impression of depth.
In Flutter, you can use the elevation
property of a Material
widget to create a raised effect. The elevation
property specifies the z-coordinate at which to place the widget, with higher values creating a more pronounced raised effect.
Most used properties of Flutter ElevatedButton
- child: will be a text or another widget
- onPressed: do something or call a function
- onLongPress: detect long press and will do something or call function
- enabled: uses a boolean value (true or false), it enables or disables the button
- style: style of the button ElevatedButton.styleFrom()
- backgroundColor: the colour of the button
- foregroundColor: the colour of the foreground elements like text or icon
- shadowColor: the colour of the buttons shadow
- elevation: elevation of the button, bigger elevation = bigger shadow
- side: border side i.e. BorderSide(color: Colors.blue, width: 3),
- shape: the shape of the button
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(30))
),
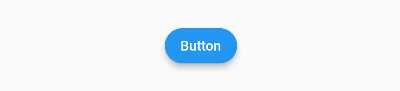
The full list of Flutter ElevatedButton.styleFrom() properties
- foregroundColor,
- backgroundColor,
- disabledForegroundColor,
- disabledBackgroundColor,
- shadowColor,
- surfaceTintColor,
- elevation,
- textStyle,
- padding,
- minimumSize,
- fixedSize,
- maximumSize,
- side,
- shape,
- enabledMouseCursor,
- disabledMouseCursor,
- visualDensity,
- tapTargetSize,
- animationDuration,
- enableFeedback,
- alignment,
- splashFactory,
- disabledForegroundColor,
- disabledBackgroundColor
How to change the size of the Flutter ElevatedButton
Use SizedBox with ElevatedButton in Flutter
You can use a SizedBox
widget to set the size of the button. The SizedBox
widget allows you to specify a specific size for its child widget. Here’s an example of how you can use the SizedBox
widget to set the size of the ElevatedButton
:
SizedBox(
width: 200.0,
height: 100.0,
child: ElevatedButton(
onPressed: (){},
onLongPress: () {
print('Long press');
},
child: const Text('flutterassets.com'),
),
),
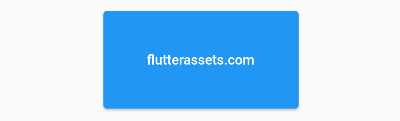
Use Container with ElevatedButton in Flutter
You can also use a Container
widget instead of a SizedBox
to set the size of an ElevatedButton
in Flutter, you can specify the height
and width
properties of the Container
widget. Here’s an example of how you can use the Container
widget to set the size of the ElevatedButton
:
Container(
width: 200.0,
height: 100.0,
child: ElevatedButton(
onPressed: (){},
onLongPress: () {
print('Long press');
},
child: const Text('flutterassets.com'),
),
),
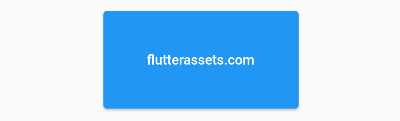
Keep in mind that the Container
widget will take up all available space if its size is not explicitly set, so it’s important to specify the height
and width
properties to set the size of the button.
You can also use the ConstrainedBox
widget to set the size of the button. The ConstrainedBox
widget allows you to specify constraints on the size of its child widget. Here’s an example of how you can use the ConstrainedBox
widget to set the size of the ElevatedButton
:
ConstrainedBox(
constraints: BoxConstraints(
minHeight: 48,
maxHeight: 64,
minWidth: 128,
maxWidth: 256,
),
child: ElevatedButton(
onPressed: () {
// Perform some action when the button is pressed
},
child: Text('Press me'),
),
),
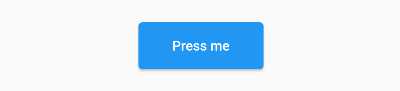
This will ensure that the ElevatedButton
is always at least 48 pixels tall and 128 pixels wide, but will not exceed those sizes.
How to disable the Flutter ElevatedButton
To disable an ElevatedButton
in Flutter, you can set the onPressed
property to null
.
ElevatedButton(
onPressed: null,
child: const Text('flutterassets.com'),
),
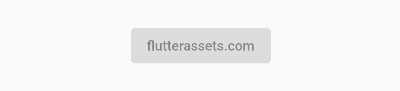
Alternatively, you can also use the AbsorbPointer
widget to disable the button. This widget absorbs all touch events that pass through it, effectively disabling the button.
Here’s an example of how you can use the AbsorbPointer
widget to disable the button:
AbsorbPointer(
absorbing: true,
child: ElevatedButton(
onPressed: () {
// button pressed event
},
child: Text('Button'),
),
)
Flutter ElevatedButton background colour
If you want to customize the background and foreground colours of an ElevatedButton in Flutter you can use the backgroundColor
and foregroundColor
properties of the style
property.
Here’s an example of how you might use the backgroundColor
and foregroundColor
properties to customize the colours of an ElevatedButton:
ElevatedButton(
onPressed: () {
// Perform some action
},
child: Text('Button'),
style: ElevatedButton.styleFrom(
backgroundColor: Colors.green,
foregroundColor: Colors.black,
),
)

In this example, the button has a green background and black text. You can use any colours that you like for the backgroundColor
and foregroundColor
properties.
Note that the foregroundColor
property controls the colour of the button’s text and icon. If you want to customize the text colour separately from the icon colour, you can use the textStyle
property of the style
property to specify a custom TextStyle
for the button’s text.
How to use ElevatedButton.icon in Flutter
To use an ElevatedButton with an icon in Flutter, you can use the ElevatedButton.icon
constructor. This constructor allows you to specify an icon to display on the button as well as text to display next to the icon.
Here’s an example of how you might use ElevatedButton.icon
in Flutter:
ElevatedButton.icon(
onPressed: (){
// Perform some action
},
icon: Icon(Icons.web_asset),
label: Text('go to flutterassets.com')
)

In this example, the button displays an web_asset
icon and the text “go to flutterassets.com” next to the icon. When the user clicks the button, an action is performed.
You can customize the appearance and behaviour of the ElevatedButton by setting various properties such as onPressed, colour, elevation, and others. For example, you can set the colour property to change the background colour of the button, or you can set the elevation
property to change the shadow depth of the button.
Flutter ElevatedButton with rounded border

In this example, I used button elevation, shadow, border, text style, padding, border radius.
ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.red, // background
foregroundColor: Colors.white, // foreground
shadowColor: Colors.blue,
elevation: 10,
side: BorderSide(color: Colors.blue, width: 3),
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(30))
),
textStyle: const TextStyle(
color: Colors.white,
fontSize: 30,
fontWeight: FontWeight.w800,
fontStyle: FontStyle.italic
),
),
onPressed: () { },
child: const Padding(
padding: EdgeInsets.all(8.0),
child: Text('Elevated Button'),
),
),
The style
property of the ElevatedButton
is used to specify the button’s visual appearance, including its background colour, foreground colour, shadow colour, elevation, border, and text style. The shape
property is used to specify the button’s border shape, and in this case, it is set to a rounded rectangle with a radius of 30 pixels.
The child
property specifies the widget that is displayed inside the button, and in this case, it is a text widget with the text “Elevated Button”. The onPressed
property specifies a callback function that is executed when the button is pressed.
onPressed and onLongPress in Flutter
In Flutter, onPressed
and onLongPress
are callback functions that can be passed as arguments to certain types of buttons, such as FlatButton
, ElevatedButton
, and IconButton
. These callback functions are called when the respective button is pressed or long-pressed by the user.
ElevatedButton(
onPressed: (){
// Perform some action when the button is pressed
},
onLongPress: () {
// Perform some action when the button is long-pressed
print('Long press');
},
child: const Text('flutterassets.com'),
),
In this example, the onPressed
callback function will be called when the user presses the button, and the onLongPress
callback function will be called when the user long-presses the button.
You can use the onPressed
and onLongPress
callback functions to specify the actions that should be performed when the button is pressed or long-pressed. For example, you can use them to trigger an API call, navigate to a new screen, or update the state of the app.
Note that the onLongPress
callback function is optional, and you don’t have to include it if you don’t need it.
Flutter ElevatedButton in a Dialog
To use an ElevatedButton in a Dialog in Flutter, you can create the Dialog using the AlertDialog
widget and include the ElevatedButton in the actions
parameter. The actions
parameter should be a list of widgets to display at the bottom of the dialog.
Here’s an example of how you might use an ElevatedButton in a Dialog in Flutter:
Column(
children: [
Center(
child: ElevatedButton(
child: Text('Show Dialog'),
onPressed: () {
showDialog(
context: context,
builder: (context) {
return AlertDialog(
title: Text('Confirm Action'),
content: Text('Are you sure you want to perform this action?'),
actions: [
ElevatedButton(
child: Text('Cancel'),
onPressed: () {
Navigator.of(context).pop();
},
),
ElevatedButton(
child: Text('OK'),
onPressed: () {
// Perform action
Navigator.of(context).pop();
},
),
],
);
},
);
},
),
)
],
)
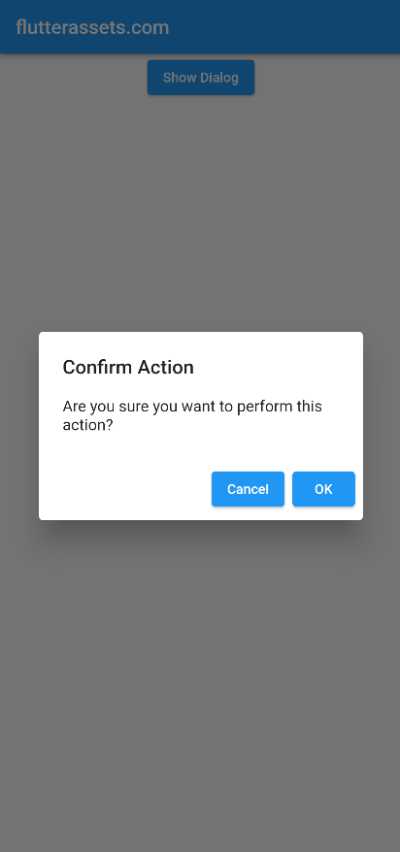
In this example, the ElevatedButton is used to display a “Cancel” button and an “OK” button in the dialog. When the user clicks the “OK” button, the action is performed and the dialog is dismissed. When the user clicks the “Cancel” button, the dialog is dismissed without performing the action.
Flutter ElevatedButton in a ListView
To create a ListView with an ElevatedButton in Flutter, you can create a ListTile for each item in the list and include the ElevatedButton as a trailing widget.
Here’s an example of how you might create a ListView with an ElevatedButton in Flutter:
final items = List<String>.generate(20, (i) => 'Item $i');
ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(items[index]),
trailing: ElevatedButton(
child: Text('Action'),
onPressed: () {
// Perform action
},
),
);
},
)
In this example, the ListView displays a list of items, and each item has an ElevatedButton as a trailing widget. When the user clicks the button, an action can be performed. You can customize the appearance and behaviour of the ElevatedButton by setting various properties such as text
, onPressed
, color
, and elevation
.
You can read more about Buttons in this post: Flutter Basics – Different types of Flutter Buttons