What can be customized in the Flutter InputChip widget
There are various ways to customize the Flutter InputChip widget. Some of the properties that can be customized include the label text, label style, label padding, avatar, avatar background colour, avatar border colour, delete icon, delete button tooltip message, selected colour, disabled colour, background colour, elevation, and more. These properties can be used to create visually appealing and interactive input chips that meet the specific needs of the app. Additionally, InputChips can be wrapped inside other widgets such as Wrap or SingleChildScrollView to allow for better display and management of multiple chips.
Here are the InputChip properties:
InputChip InputChip({
Key? key,
Widget? avatar,
required Widget label,
TextStyle? labelStyle,
EdgeInsetsGeometry? labelPadding,
bool selected = false,
bool isEnabled = true,
void Function(bool)? onSelected,
Widget? deleteIcon,
void Function()? onDeleted,
Color? deleteIconColor,
String? deleteButtonTooltipMessage,
void Function()? onPressed,
double? pressElevation,
Color? disabledColor,
Color? selectedColor,
String? tooltip,
BorderSide? side,
OutlinedBorder? shape,
Clip clipBehavior = Clip.none,
FocusNode? focusNode,
bool autofocus = false,
Color? backgroundColor,
EdgeInsetsGeometry? padding,
VisualDensity? visualDensity,
MaterialTapTargetSize? materialTapTargetSize,
double? elevation,
Color? shadowColor,
Color? surfaceTintColor,
IconThemeData? iconTheme,
Color? selectedShadowColor,
bool? showCheckmark,
Color? checkmarkColor,
ShapeBorder avatarBorder = const CircleBorder(),
bool useDeleteButtonTooltip = true,
})
How to use an avatar in Flutter InputChip
The avatar property is used to display an image or icon that represents the InputChip
. This can be useful for displaying a user’s profile picture or a tag icon. You can set the avatar property to any widget that represents an image or icon.
Here is an example of how to use the avatar property in a InputChip
:
InputChip(
avatar: CircleAvatar(
backgroundImage: NetworkImage('https://picsum.photos/250?image=9'),
),
label: Text('flutterassets.com'),
onSelected: (isSelected) {},
),

In this example, we set the InputChip
avatar property to a CircleAvatar
widget. The CircleAvatar
widget displays an image that represents the user’s profile picture, which is loaded from a network image URL. The label
property is set to the user’s name, which will be displayed next to the avatar image.
When the InputChip
is selected, the onSelected
callback is called, which allows you to handle the chip selection as needed.
Flutter InputChip widget avatar with Image
To use an asset image as the avatar in an InputChip
, you can use the Image.asset
widget as the child of a CircleAvatar
widget, like this:
InputChip(
avatar: CircleAvatar(
child: Image.asset('assets/logo.png'),
),
label: Text('flutterassets.com'),
onSelected: (isSelected) {},
),
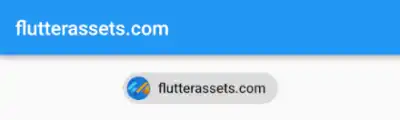
In this example, we set the InputChip
avatar property to a CircleAvatar
widget that contains an Image.asset
widget as its child. The Image.asset
widget displays an image that is stored in the app’s assets folder. The label
property is set to the chip’s label text, which will be displayed next to the avatar image.
Flutter InputChip widget avatar with Icon
To use an icon as the avatar in an InputChip
, you can use the Icon
widget as the child of a CircleAvatar
widget, like this:
InputChip(
avatar: CircleAvatar(
child: Icon(Icons.person),
),
label: Text('flutterassets.com'),
onSelected: (isSelected) {},
),

In this example, we set the InputChip
avatar property to a CircleAvatar
widget that contains an Icon
widget as its child. The Icon
widget displays a label icon. The label
property is set to the chip’s label text, which will be displayed next to the avatar icon.
How to customize the colours of the InputChip in Flutter
The InputChip
widget has several colour properties that can be customized to style the InputChip
widget, including deleteIconColor
, disabledColor
, selectedColor
, backgroundColor
, shadowColor
, surfaceTintColor
, selectedShadowColor
, and checkmarkColor
.
Here’s an example of how to use these colour properties to customize the InputChip
widget:
InputChip(
label: Text('flutterassets.com'),
onSelected: (isSelected) {},
deleteIconColor: Colors.red,
disabledColor: Colors.grey,
selectedColor: Colors.blue,
backgroundColor: Colors.blue.shade100,
shadowColor: Colors.grey,
surfaceTintColor: Colors.grey[200],
selectedShadowColor: Colors.blue,
checkmarkColor: Colors.white,
),
Let’s describe each of the colour properties used in this example:
deleteIconColor
: This property sets the colour of the delete icon that appears on the right side of the chip when it is deletable. In this example, we set it toColors.red
.disabledColor
: This property sets the background colour of the chip when it is disabled. In this example, we set it toColors.grey
.selectedColor
: This property sets the background colour of the chip when it is selected. In this example, we set it toColors.blue
.backgroundColor
: This property sets the background colour of the chip when it is not selected or disabled. In this example, we set it toColors.blue.shade100
.shadowColor
: This property sets the colour of the shadow that appears around the chip. In this example, we set it toColors.grey
.surfaceTintColor
: This property sets the colour of the surface that the chip is on, which affects the chip’s shadow colour. In this example, we set it toColors.grey[200]
.selectedShadowColor
: This property sets the colour of the shadow that appears around the chip when it is selected. In this example, we set it toColors.blue
.checkmarkColor
: This property sets the colour of the checkmark that appears on the left side of the chip when it is selected. In this example, we set it toColors.white
.
How to use onSelected in Flutter InputChip
The onSelected
property in Flutter’s InputChip
widget is a callback that is triggered when the chip is selected or unselected. It takes a function that accepts a boolean value, which represents whether the chip is currently selected or not.
Here’s an example of how to use the onSelected
property in Flutter’s InputChip
widget:
bool _isSelected = false;
InputChip(
label: Text('flutterassets.com'),
selected: _isSelected,
onSelected: (isSelected) {
setState(() {
_isSelected = isSelected;
});
},
),
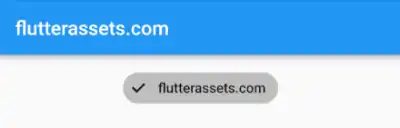
In this example, we first declare a boolean variable _isSelected
to keep track of whether the chip is selected or not. We then create an InputChip
widget with a label of “flutterassets.com”, set the initial selection state to _isSelected
, and pass a function to the onSelected
property.
Inside the function passed to onSelected
, we use setState
to update the value of _isSelected
to match the new selection state. This ensures that the UI is updated to reflect the new state of the chip.
When the user taps the chip, the onSelected
function is called with a boolean value that represents the new selection state. In this example, we simply update the _isSelected
variable to match the new state, but you could also perform other actions based on the selection state if needed.
How to use onDeleted in Flutter InputChip
The onDeleted
property in Flutter’s InputChip
widget is a callback that is triggered when the delete icon is pressed.
Here’s an example of how to use the onDeleted
property in Flutter’s InputChip
widget:
InputChip(
label: Text('flutterassets.com'),
deleteIcon: Icon(Icons.clear),
onDeleted: () {
// Perform actions when the delete icon is pressed
},
),
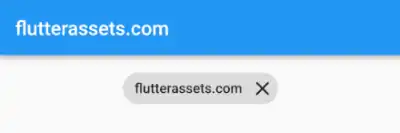
In this example, we create an InputChip
widget with a label of “flutterassets.com” and a delete icon of the clear icon. We pass a function to the onDeleted
property, which will be called when the delete icon is pressed.
Inside the function passed to onDeleted
, you can perform any custom actions that you need, such as removing the chip from a list or performing an API call to update the data.
It’s worth mentioning that if the onDeleted
property is not set, the delete icon will not be displayed on the chip.
How to use onPressed in Flutter InputChip
The onPressed
property in Flutter’s InputChip
widget is a callback that is triggered when the chip is pressed.
Here’s an example of how to use the onPressed
property in Flutter’s InputChip
widget:
InputChip(
label: Text('flutterassets.com'),
onPressed: () {
// Perform actions when the chip is pressed
},
),
In this example, we create an InputChip
widget with a label of “flutterassets.com” and set the onPressed
property to a function that will be called when the chip is pressed.
Inside the function passed to onPressed
, you can perform any custom actions that you need, such as opening a new screen or displaying a dialog.
It’s worth mentioning that the onPressed
property is not related to the chip’s selection state. If you need to perform custom actions based on whether the chip is selected or not, you should use the onSelected
property instead.
How to customize the Label in the Flutter InputChip widget
In Flutter’s InputChip
widget, you can customize the label in several ways to better suit your app’s design. Here are a few customization options for the label with code examples:
Changing the InputChip label text style:
InputChip(
label: Text('flutterassets.com',
style: TextStyle(
fontWeight: FontWeight.bold,
fontStyle: FontStyle.italic,
color: Colors.white,
),
),
),
In this example, we set the fontWeight
property to FontWeight.bold
and the fontStyle
property to FontStyle.italic
in the TextStyle
object. We also set the text colour to white using the color
property.
Changing the InputChip label padding:
InputChip(
label: Text('flutterassets.com'),
labelPadding: EdgeInsets.symmetric(vertical: 10.0, horizontal: 16.0),
),
In this example, we set the labelPadding
property to add some padding around the label text. We use the EdgeInsets.symmetric
method to specify the amount of padding for the vertical and horizontal directions.
Adding an icon to the label in InputChip:
InputChip(
label: Row(
children: [
Icon(Icons.star, color: Colors.red),
SizedBox(width: 14.0),
Text('flutterassets.com'),
],
),
onSelected: (selected){},
),
In this example, we use a Row
widget to add an icon to the label. We first add an Icon
widget with the Icons.star
icon and set its colour using the color
property. We then add a SizedBox
widget to create some space between the icon and the text. Finally, we add a Text
widget with the label text.
Changing the InputChip label background colour:
InputChip(
label: Text('flutterassets.com'),
backgroundColor: Colors.lightGreenAccent,
onSelected: (selected){},
),
In this example, we set the backgroundColor
property to change the background colour of the chip.
How to use selected in the Flutter InputChip
The selected
property in the Flutter InputChip
widget is used to determine whether the chip is currently selected or not. When the selected
property is set to true
, the chip is highlighted and the background colour changes to the selectedColor
.
To use the selected
property, you can define a boolean variable to keep track of whether the chip is selected or not. You can then use this variable to set the selected
property of the InputChip
.
Here’s an example code that demonstrates how to use the selected
property in a InputChip
:
bool _isSelected = false;
InputChip(
label: Text('flutterassets.com'),
selected: _isSelected,
selectedColor: Colors.blue,
onSelected: (bool selected) {
setState(() {
_isSelected = selected;
});
},
),
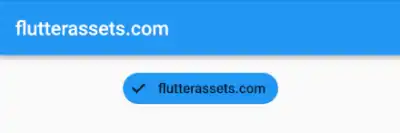
In this example, we have defined a boolean variable _isSelected
which keeps track of whether the chip is selected or not. The InputChip
widget is then created with the label
set to Text('flutterassets.com')
, selected
set to _isSelected
, and selectedColor
set to Colors.blue
. The onSelected
callback is also defined, which is called when the chip is selected or unselected. Inside the onSelected
callback, we update the _isSelected
variable and call setState()
to rebuild the widget tree with the new selection state.
By default, the selected
property is set to false
, which means the chip is not selected. When the chip is selected, the _isSelected
variable is updated and the InputChip
widget is rebuilt with the new selection state, which causes the background colour to change to the selectedColor
.
How to use isEnabled in the Flutter InputChip
The isEnabled
property in the Flutter InputChip
widget is used to enable or disable the chip. When the isEnabled
property is set to false
, the chip is disabled and cannot be selected or deleted.
To use the isEnabled
property, you can define a boolean variable to keep track of whether the chip is enabled or not. You can then use this variable to set the isEnabled
property of the InputChip
.
Here’s an example code that demonstrates how to use the isEnabled
property in a InputChip
:
bool _isEnabled = false;
InputChip(
label: Text('flutterassets.com'),
isEnabled: _isEnabled,
onSelected: (bool selected) {
// Your code here
},
onDeleted: () {
// Your code here
},
),
In this example, we have defined a boolean variable _isEnabled
which keeps track of whether the chip is enabled or not. The InputChip
widget is then created with the label
set to Text('flutterassets.com')
and isEnabled
set to _isEnabled
. The onSelected
and onDeleted
callbacks are also defined, which are called when the chip is selected or deleted, respectively.
By default, the isEnabled
property is set to true
, which means the chip is enabled. When the _isEnabled
variable is set to false
, the chip is disabled and cannot be selected or deleted. When the _isEnabled
variable is set to true
, the chip is enabled and can be selected or deleted as usual.
How to change the shape of the Flutter InputChip
In Flutter, the shape of an InputChip
can be customized using the shape
property. The shape
property takes an instance of the ShapeBorder
class, which can be used to define the shape of the chip.
There are five built-in shapes available in Flutter:
StadiumBorder
: This creates a chip with rounded edges.RoundedRectangleBorder
: This creates a chip with rounded corners.BeveledRectangleBorder
: This creates a chip with bevelled edges.ContinuousRectangleBorder
: This creates a chip with straight edges.CircleBorder
: This creates a circular chip.
Here’s an example code that demonstrates how to use these shapes:
Column(
children: [
InputChip(
label: Text('Stadium Border'),
shape: StadiumBorder(),
),
InputChip(
label: Text('Rounded Rectangle Border'),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
),
),
InputChip(
label: Text('Beveled Rectangle Border'),
shape: BeveledRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
),
InputChip(
label: Text('Continuous Rectangle Border'),
shape: ContinuousRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
),
),
InputChip(
label: Text('Continuous Rectangle Border'),
shape: ContinuousRectangleBorder(),
),
InputChip(
label: Text('Circle Border'),
shape: CircleBorder(),
),
InputChip(
label: Text('Circle Border'),
padding: EdgeInsets.symmetric(vertical: 40),
shape: CircleBorder(),
),
],
)

In this example, we have defined five InputChip
widgets, each with a different shape. The first chip has a StadiumBorder
shape, the second has a RoundedRectangleBorder
shape, the third has a BeveledRectangleBorder
shape, the fourth has a ContinuousRectangleBorder
shape, and the fifth has a CircleBorder
shape.
To use a specific shape, we need to create an instance of the corresponding ShapeBorder
subclass and pass it to the shape
property of the InputChip
. We can also customize the shape by passing different parameters to the constructor of the ShapeBorder
subclass. In the case of the RoundedRectangleBorder
and BeveledRectangleBorder
, we need to pass a BorderRadius
object to the constructor to define the radius of the corners.
How to add a border to the Flutter InputChip
To add a black border to the Flutter InputChip, we can use the side
property of the InputChip
widget. We can set the side
property to a BorderSide
object with the desired colour and width of the border.
Here’s an example code to add a black border to an InputChip
with each of the 5 different shapes available in Flutter:
Column(
children: [
InputChip(
label: Text('Stadium Border'),
shape: StadiumBorder(
side: BorderSide(color: Colors.black, width: 2.0),
),
),
InputChip(
label: Text('Rounded Rectangle Border'),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
side: BorderSide(color: Colors.black, width: 2.0),
),
),
InputChip(
label: Text('Beveled Rectangle Border'),
shape: BeveledRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
side: BorderSide(color: Colors.black, width: 2.0),
),
),
InputChip(
label: Text('Continuous Rectangle Border'),
shape: ContinuousRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
side: BorderSide(color: Colors.black, width: 2.0),
),
),
InputChip(
label: Text('Continuous Rectangle Border'),
shape: ContinuousRectangleBorder(
side: BorderSide(color: Colors.black, width: 2.0),
),
),
InputChip(
label: Text('Circle Border'),
shape: CircleBorder(
side: BorderSide(color: Colors.black, width: 2.0),
),
),
InputChip(
label: Text('Circle Border'),
padding: EdgeInsets.symmetric(vertical: 40),
shape: CircleBorder(
side: BorderSide(color: Colors.black, width: 2.0),
),
),
],
)
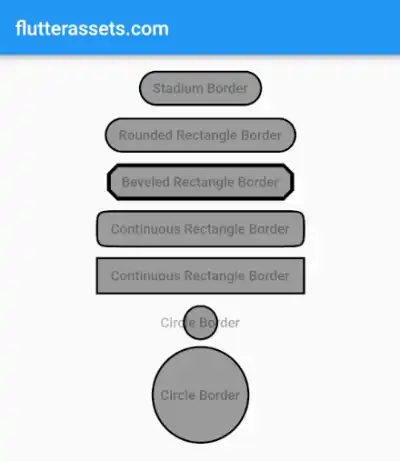
In the above example, we’ve set the side
property of each shape’s border to a BorderSide
object with a black colour and a width of 2. This creates a thin black border around the InputChip
.