The Flutter BoxFit
class is a set of options that determines how an image should be resized and positioned inside a container. It’s commonly used with the Image
widget to control how the image appears within a specific area. The BoxFit
class has several values that you can use to specify how the image should be displayed.
These values include: fill
, contain
, cover
, fitHeight
, fitWidth
, scaleDown
, and none
. Each value changes the size and placement of the image in a slightly different way. You can choose the value that works best for your app based on the size and shape of the container and the image you want to display.
How to use BoxFit in Flutter
To use the Flutter BoxFit
class, you’ll need to set the fit
property of an Image
widget. The fit
property determines how the image should be resized and positioned within the container.
Here’s an example of how you might use BoxFit
in a Flutter app:
Image.network('https://picsum.photos/250?image=9',
fit: BoxFit.cover,
)
In this example, the BoxFit.cover
value is used to resize and position the image. This value will scale the image as large as possible while still covering the entire container and preserving the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container, some parts of the image may be cropped to fill the container.
You can use any of the other BoxFit
values in the same way. Simply set the fit
property to the value that you want to use.
How to stretch an image to fit the background in Flutter

To stretch an image to fit the background in Flutter, you can use the BoxFit.fill
value in the fit
property of an Image
widget. This will stretch the image to fill the entire container, potentially distorting the aspect ratio of the image.
Here’s an example of how you might use BoxFit.fill
and BoxFit.cover
:
Column(
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
Container(
width: MediaQuery.of(context).size.width,
height: 100,
decoration: BoxDecoration(
image: DecorationImage(
fit: BoxFit.fill,
image: AssetImage('assets/FlutterAssets_logo_BG.jpg')
),
),
),Container(
width: MediaQuery.of(context).size.width,
height: 100,
decoration: BoxDecoration(
image: DecorationImage(
fit: BoxFit.cover,
image: AssetImage('assets/FlutterAssets_logo_BG.jpg')
),
),
),
],
),
Use Flutter fit BoxFit options
Here are the different options available for BoxFit
:
source: Images and text from https://api.flutter.dev/flutter/painting/BoxFit-class.html.
Use BoxFit contain in Flutter
Scales the image as large as possible while still fitting within the container and preserving the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container, empty space may be added to the sides or top and bottom of the image to fill the container.
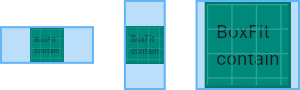
You can use this BoxDecoration
as the decoration
property of a Container
widget, for example:
Container(
width: 150,
height: 150,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.contain,
),
),
)
Row(
children: [
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.contain,
),
),
),
SizedBox(width: 10,),
Container(
width: 200,
height: 100,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.contain,
),
),
),
],
)
This will display the image within a box with a blue background colour. The image will be scaled so that it is as large as possible within the box while maintaining its aspect ratio. This may result in empty space within the box if the aspect ratio of the box does not match the aspect ratio of the image.
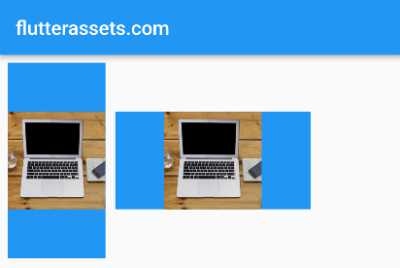
Use BoxFit cover in Flutter
Scales the image as large as possible while still covering the entire container and preserving the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container, some parts of the image may be cropped to fill the container.
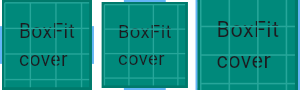
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.cover,
),
),
),
This will display the image within a box with a blue background colour. The image will be scaled to cover the entire box, while maintaining its aspect ratio. This may result in some parts of the image being cropped if the aspect ratio of the box does not match the aspect ratio of the image.
Row(
children: [
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.cover,
),
),
),
SizedBox(width: 10,),
Container(
width: 200,
height: 100,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.cover,
),
),
),
],
)

Use BoxFit fill in Flutter
Stretches the image to fill the entire container, potentially distorting the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container, some parts of the image may be cropped to fit within the container.
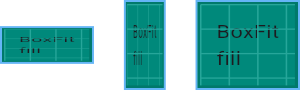
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fill,
),
),
),
Row(
children: [
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fill,
),
),
),
SizedBox(width: 10,),
Container(
width: 200,
height: 100,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fill,
),
),
),
],
)
The BoxFit.fill
option will stretch the image to fit the entire container, potentially distorting the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container.
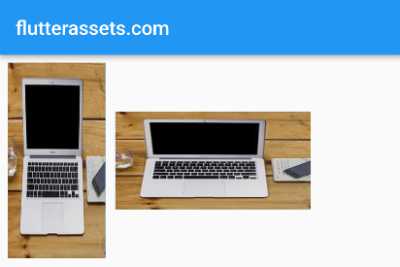
Use BoxFit fitHeight in Flutter
Stretches the image to fit the entire height of the container while preserving the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container, empty space may be added to the sides of the image to fill the container.
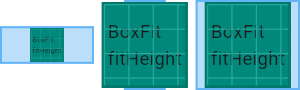
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fitHeight,
),
),
),
Row(
children: [
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fitHeight,
),
),
),
SizedBox(width: 10,),
Container(
width: 200,
height: 100,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fitHeight,
),
),
),
],
)
The BoxFit.fitHeight
option will stretch the image to fit the entire height of the container while preserving the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container, empty space may be added to the sides of the image to fill the container.

Use BoxFit fitWidth in Flutter
Stretches the image to fit the entire width of the container while preserving the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container, empty space may be added to the top and bottom of the image to fill the container.
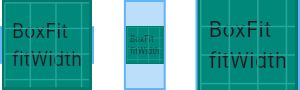
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fitWidth,
),
),
),
Row(
children: [
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fitWidth,
),
),
),
SizedBox(width: 10,),
Container(
width: 200,
height: 100,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.fitWidth,
),
),
),
],
)
The BoxFit.fitWidth
option will stretch the image to fit the entire width of the container while preserving the aspect ratio of the image. If the aspect ratio of the image does not match the aspect ratio of the container, empty space may be added to the top and bottom of the image to fill the container.

Use BoxFit scaleDown in Flutter
Scales the image down as much as possible while still showing the entire image and preserving the aspect ratio. If the aspect ratio of the image matches the aspect ratio of the container, the image will not be scaled at all. If the aspect ratio of the image does not match the aspect ratio of the container, the image may be scaled down and some parts of the container may remain empty.
This is the same as contain
if that would shrink the image, otherwise it is the same as none
.
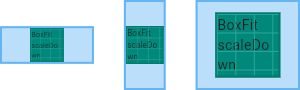
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/50?image=9'),
fit: BoxFit.scaleDown,
),
),
),
Row(
children: [
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/50?image=9'),
fit: BoxFit.scaleDown,
),
),
),
SizedBox(width: 10,),
Container(
width: 200,
height: 100,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/50?image=9'),
fit: BoxFit.scaleDown,
),
),
),
],
)
The BoxFit.scaleDown
option will scale the image down as much as possible while still showing the entire image and preserving the aspect ratio. If the aspect ratio of the image matches the aspect ratio of the container, the image will not be scaled at all. If the aspect ratio of the image does not match the aspect ratio of the container, the image may be scaled down and some parts of the container may remain empty.

Use BoxFit none in Flutter
Does not scale the image at all. The image will be displayed at its original size, and if it is larger than the container, parts of the image may be cropped. If the aspect ratio of the image does not match the aspect ratio of the container, empty space may be added to the sides or top and bottom of the image to fill the container.
The source image is not resized.
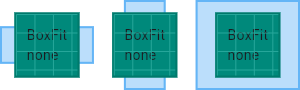
If you want to display an image without scaling or resizing it, you can use the Image
widget with the fit
property set to BoxFit.none
. This will display the image at its original size, without scaling or resizing it.
Image(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.none,
)
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.none,
),
),
),
Row(
children: [
Container(
width: 100,
height: 200,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.none,
),
),
),
SizedBox(width: 10,),
Container(
width: 200,
height: 100,
decoration: BoxDecoration(
color: Colors.blue,
image: DecorationImage(
image: NetworkImage('https://picsum.photos/250?image=9'),
fit: BoxFit.none,
),
),
),
],
)
The BoxFit.none
option will not scale the image at all. The image will be displayed at its original size, and if it is larger than the container, parts of the image may be cropped. If the aspect ratio of the image does not match the aspect ratio of the container, empty space may be added to the sides or top and bottom of the image to fill the container.
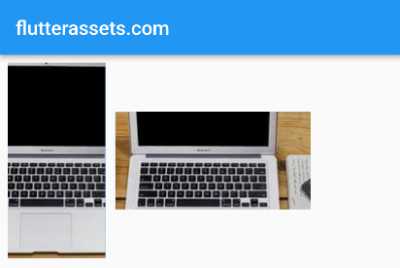
You can read more about Images in Flutter in this post: Flutter Basics – How to use an image in Flutter