The FloatingActionButton widget is often positioned in the bottom-right corner of the screen and has a circular form. It also has an elevation to provide the illusion of floating. The best part is that all of these features are fully configurable.
Here are the properties of this button.
- autofocus: uses a boolean value (true or false)
- backgroundColor: background colour of the button
- child: here is the widget, like icon, image, or text that will be displayed.
- elevation: uses the double value and controls the button elevation (z-coordinate) adding a bit of shadow under
- disabledElevation: uses values as above but for the disabled button
- focusColor: colour when the button is in focus – i.e. Colors.lightGreenAccent
- focusElevtion: elevation when the button is in focus
- focusNode: It provides an additional focus node for the button.
- foregroundColor: the colour of stuff inside the button like icon or text
- heorTag: add a hero tag to one of the widgets in the button
- highlightElevation: it changes the elevation when you interact with the button like click
- hoverColor: colour of the button at the event of hover
- hoverElevation: elevation of the button at the event of hover
- isExtended: use the boolean value. If the value is true the button will be extended i.e. with text. I will add the example below.
- materialTapTargetSize: This property is there to control the tap target size of the button
- mini: the mini size of the button, see example below
- mouseCursor: This property accepts the MouseCursor property as an object. When it interacts with the button, it controls the mouse pointer’s cursor.
- onPressed: do something or call function
- splashColor: splash colour of FloatingActionButton in other words colour when you press the button
- shape: the shape of the button. example below
How to disable the FloatingActionButton in Flutter
To disable a FloatingActionButton
in Flutter, you can set its onPressed
property to null
. This will prevent the button from being clicked and will also cause it to be displayed as disabled, with a lower opacity. Here’s an example of how you might do this:
FloatingActionButton.extended(
onPressed: null,
isExtended: true,
label: const Text('Home'),
icon: const Icon(
Icons.home_outlined
),
)
Here is another quick simple example:
FloatingActionButton(
onPressed: null, // disable the button
child: Icon(Icons.add),
)
How to use FloatingActionButton.extended in Flutter
FloatingActionButton.extended
is a variant of the FloatingActionButton
widget that allows you to display a button with a label or additional content. So simply say, you can extend the size of the button and add text inside. The button has the isExtended property which can turn the
ON or OFF the extension ( hide the label). It uses the value true or false.
FloatingActionButton.extended(
onPressed: () {},
isExtended: true,
label: const Text('Home'),
icon: const Icon(
Icons.home_outlined
),
)

How to change the shape of FloatingActionButton in Flutter
To change the shape of a FloatingActionButton
in Flutter, you can use the shape
property. This property allows you to specify a ShapeBorder
object that defines the shape of the button.
Here’s an example of how you might use the shape
property to create a circular FloatingActionButton
:
floatingActionButton: FloatingActionButton(
onPressed: () {
// handle the button press here
},
child: Icon(Icons.add),
shape: const CircleBorder(
side: BorderSide(width: 2,color: Colors.red)
), // specify a CircleBorder shape
),
You can also create more complex shapes by using the RoundedRectangleBorder
class. Here’s an example of how you might use it to create a FloatingActionButton
with rounded corners:
FloatingActionButton.extended(
onPressed: () {},
isExtended: true,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(5.0),
),
label: const Text('Home'),
icon: const Icon(
Icons.home_outlined
),
)
FloatingActionButton.extended(
onPressed: () {},
materialTapTargetSize: MaterialTapTargetSize.padded,
isExtended: true,
splashColor: Colors.lightGreenAccent,
shape: RoundedRectangleBorder(
side: BorderSide(color: Colors.red, width: 3),
borderRadius: BorderRadius.circular(10),
),
label: const Text('Vilage'),
icon: const Icon(
Icons.holiday_village_outlined
),
),
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(
Radius.circular(8.0),
),
),

Where in the Flutter code the FloatingActionButton should be
The FloatingActionButton
should be placed within the floatingActionButton
property of the Scaffold
widget. The Scaffold
is a container that provides a common structure for app bars, drawers, and the FloatingActionButton
.
Here’s an example of how you might use the FloatingActionButton
in a Flutter app:
Scaffold(
appBar: AppBar(
title: Text('flutterassets.com'),
),
body: Center(
child: Text('Hello World'),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
// handle the button press here
},
child: Icon(Icons.add),
),
)
In this example, the FloatingActionButton
will be displayed in the bottom right corner of the screen and will execute the onPressed
callback when clicked.
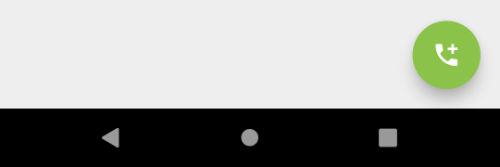
FloatingActionButton regular size (56×56)
The regular size of the button is the one that is mostly used in app development. Every time when you start a new project in the android studio you will see this button in the template project.
floatingActionButton: FloatingActionButton(
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
FloatingActionButton small(40×40) or mini = true
You can specify the size of the button using the mini
property. If set to true
, the button will have a mini size, which is smaller than the regular size.
Here’s an example of a regular-sized FloatingActionButton
:
FloatingActionButton(
onPressed: () {
// handle the button press here
},
child: Icon(Icons.add),
mini: true, // mini size
)
You can also use FloatingActionButton.small
:
floatingActionButton: FloatingActionButton.small(
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
//mini = true;
floatingActionButton: FloatingActionButton(
mini: true,
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
FloatingActionButton large
You can create a large circular floating action button using the FloatingActionButton.large
.
floatingActionButton: FloatingActionButton.large(
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
Here you have a slightly modified FloatingActionButton
floatingActionButton: SizedBox(
height: 100.0,
width: 100.0,
child: FittedBox(
child: FloatingActionButton(
backgroundColor: Colors.lightGreen,
elevation: 10,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),
),
),
Two FloatingActionButtons in a column or a row
The floatingActionButton attribute on the Scaffold widget does not have to take a FloatingActionButton widget. It also accepts Column and Row widgets.
My Scaffold widget example with two floating action buttons in the column is shown below.
floatingActionButton: Column(
mainAxisAlignment: MainAxisAlignment.end,
children: [
FloatingActionButton(
onPressed: () {},
child: const Icon(
Icons.delete
),
),
const SizedBox(
height: 10,
),
FloatingActionButton(
onPressed: () {},
child: const Icon(
Icons.star
),
)
]
)

I also added the example of two floatingActionButton in a row.
floatingActionButton: Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
FloatingActionButton(
onPressed: () {},
child: const Icon(
Icons.holiday_village_outlined
),
),
const SizedBox(
width: 10,
),
FloatingActionButton(
onPressed: () {},
child: const Icon(
Icons.home_outlined
),
)
]
)

FloatingActionButton custom positions
The position of the FloatingActionButton
is determined by the floatingActionButtonLocation
property of the Scaffold
widget. The Scaffold
is a container that provides a common structure for app bars, drawers, and the FloatingActionButton
.
By default, the FloatingActionButton
is displayed in the bottom right corner of the screen. You can change this by setting the floatingActionButtonLocation
property of the Scaffold
to a different value. For example, to position the FloatingActionButton
in the bottom left corner of the screen, you can use the FloatingActionButtonLocation.endFloat
value like this:
floatingActionButton: FloatingActionButton(
onPressed: () {
// handle the button press here
},
child: Icon(Icons.add),
),
floatingActionButtonLocation: FloatingActionButtonLocation.endFloat, // position in bottom left corner
You can also use the FloatingActionButtonLocation.centerDocked
value to position the FloatingActionButton
in the centre of the screen, docked to the bottom of the screen:
floatingActionButton: FloatingActionButton(
onPressed: () {
// handle the button press here
},
child: Icon(Icons.add),
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked, // position in center, docked to bottom
Here you have predefined floatingActionButton positions
- floatingActionButtonLocation: FloatingActionButtonLocation.startDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.centerTop,
- floatingActionButtonLocation: FloatingActionButtonLocation.endDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.endFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.endTop,
- floatingActionButtonLocation: FloatingActionButtonLocation.startFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.startTop,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterTop,
- loatingActionButtonLocation: FloatingActionButtonLocation.miniEndDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniEndFloat,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniEndTop,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniStartDocked,
- floatingActionButtonLocation: FloatingActionButtonLocation.miniStartFloat
- floatingActionButtonLocation: FloatingActionButtonLocation.miniStartTop
floatingActionButtonLocation: FloatingActionButtonLocation.miniStartFloat,
floatingActionButton: FloatingActionButton(
elevation: 10,
backgroundColor: Colors.lightGreen,
onPressed: () {},
tooltip: 'add call',
child: const Icon(Icons.add_call),
),