What is a TextStyles in Flutter
In Flutter, the Text
widget allows you to style your text with a rich set of visual and control-based styling options. You can set the text colour, font size, font weight, font family, and much more. You can also use rich text formatting, such as bold, italic, and underline, and you can even apply custom text styles using a TextStyle
object.
To create a TextStyle
object, you can use the TextStyle
constructor and pass in your desired styling options as arguments. For example:
TextStyle myTextStyle = TextStyle(
fontSize: 24,
color: Colors.black,
fontWeight: FontWeight.w500,
fontStyle: FontStyle.italic,
);
You can then use this TextStyle
object to style a Text
widget by passing it to the style
property:
Text(
'My styled text',
style: myTextStyle,
)
You can also use the DefaultTextStyle
widget to apply a default text style to a whole subtree of widgets. This can be useful if you want to ensure a consistent text style throughout your app.
What is Flutter Text.rich
Certainly! Text.rich
is a widget in Flutter that allows you to display a paragraph of mixed-style text. It is built on top of the RichText
widget and allows you to specify a different TextStyle
for each span of text within the paragraph.
Using Text.rich
, you can create a paragraph of text that has multiple styles applied to it, such as different font sizes, colours, and weights. This can be useful for creating complex layouts that require a mix of text styles, or for highlighting certain words or phrases within a paragraph.
How to use multiple Text styles in Flutter
To use multiple text styles in Flutter using Text.rich
, you can create a TextSpan
widget for each span of text that you want to style differently, and specify the desired TextStyle
for each TextSpan
. You can then pass a list of TextSpan
widgets as the children
property of a TextSpan
widget, which you can pass to the text
property of the Text.rich
widget.
Here’s an example of how you can use Text.rich
to display a paragraph with different text styles:
Text.rich(
TextSpan(
children: [
TextSpan(text: 'This text is '),
TextSpan(text: 'bold', style: TextStyle(fontWeight: FontWeight.bold)),
TextSpan(text: ' and this text is ', style: TextStyle(fontSize: 24)),
TextSpan(text: 'italic', style: TextStyle(fontStyle: FontStyle.italic)),
],
),
)

In this example, the first span of text is styled using the default text style, the second span is styled with a bold font weight, the third span with a font size of 24, and the fourth span are styled with the default style and italic font style, respectively.
You can read more about the Text widget in this post: Flutter Basics – What is and how to use Text widget in Flutter
You can also specify different text styles for individual words or characters within a span of text by creating nested TextSpan
widgets. For example:
Text.rich(
TextSpan(
text: '\"',
style: TextStyle(
fontSize: 30,
color: Colors.red,
fontFamily: "Orbitron",
fontWeight: FontWeight.w900,
),
children: <TextSpan>[
TextSpan(
text: 'flutterassets.',
style: TextStyle(
color: Colors.green,
fontFamily: "Orbitron",
fontWeight: FontWeight.w900,
)
),
TextSpan(
text: 'com',
style: TextStyle(
color: Colors.blue,
fontFamily: "Genos",
fontWeight: FontWeight.w900,
decoration: TextDecoration.underline,
decorationColor: Colors.red,
decorationStyle: TextDecorationStyle.dotted,
)
),
TextSpan(
text: '\"',
style: TextStyle(
fontSize: 30,
color: Colors.red,
fontFamily: "Orbitron",
fontWeight: FontWeight.w900,
),
),
],
),
),
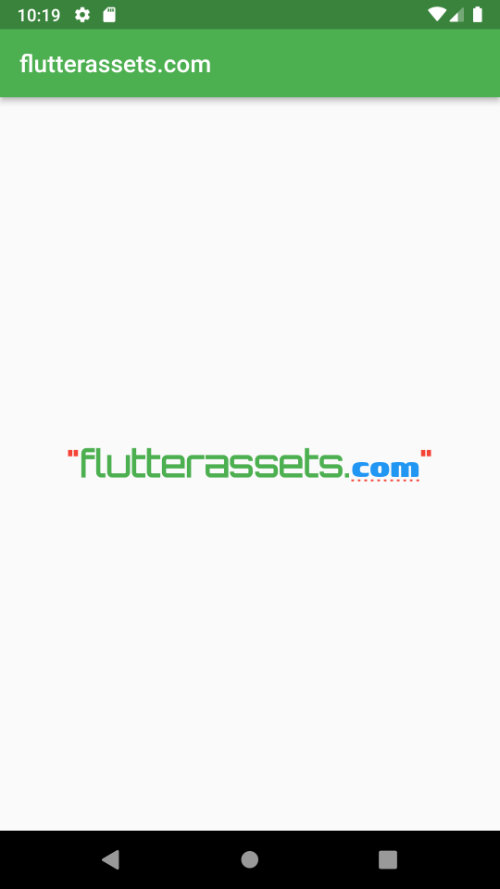
What is RichText in Flutter
RichText
is a widget in Flutter that displays a paragraph of mixed-style text. It is built on top of the TextSpan
widget, which allows you to specify a different TextStyle
for each span of text within the paragraph.
Using RichText
, you can create a paragraph of text that has multiple styles applied to it, such as different font sizes, colours, and weights. This can be useful for creating complex layouts that require a mix of text styles, or for highlighting certain words or phrases within a paragraph.
How to use RichText in Flutter
To use RichText
in Flutter, you can create a TextSpan
widget for each span of text that you want to style differently, and specify the desired TextStyle
for each TextSpan
. You can then pass a list of TextSpan
widgets as the children
property of a TextSpan
widget, which you can pass to the text
property of the RichText
widget.
Here’s an example of how you can use RichText
to display a paragraph with different text styles:
TextStyle defaultStyle = TextStyle(fontSize: 18, color: Colors.black);
TextStyle boldStyle = TextStyle(fontWeight: FontWeight.bold, color: Colors.red);
TextStyle italicStyle = TextStyle(fontStyle: FontStyle.italic, color: Colors.red);
RichText(
text: TextSpan(
children: [
TextSpan(text: 'This text is ', style: defaultStyle),
TextSpan(text: 'bold', style: boldStyle),
TextSpan(text: ' and this text is ', style: defaultStyle),
TextSpan(text: 'italic', style: italicStyle),
],
),
)


This will display the text “This is some bold and italic text.” with the word “bold” in bold and the word “italic” in italic.
Here is another example:
RichText(
text: TextSpan(
text: '\"',
style: TextStyle(
fontSize: 30,
color: Colors.red,
fontFamily: "Orbitron",
fontWeight: FontWeight.w900,
),
children: <TextSpan>[
TextSpan(
text: 'flutterassets.',
style: TextStyle(
color: Colors.green,
fontFamily: "Orbitron",
fontWeight: FontWeight.w900,
)
),
TextSpan(
text: 'com',
style: TextStyle(
color: Colors.blue,
fontFamily: "Genos",
fontWeight: FontWeight.w900,
decoration: TextDecoration.underline,
decorationColor: Colors.red,
decorationStyle: TextDecorationStyle.dotted,
)
),
TextSpan(
text: '\"',
style: TextStyle(
fontSize: 30,
color: Colors.red,
fontFamily: "Orbitron",
fontWeight: FontWeight.w900,
),
),
],
),
),
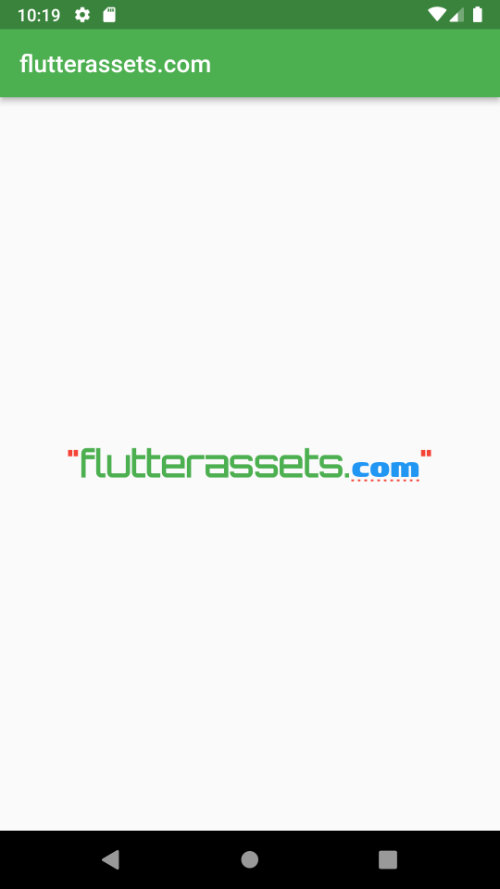
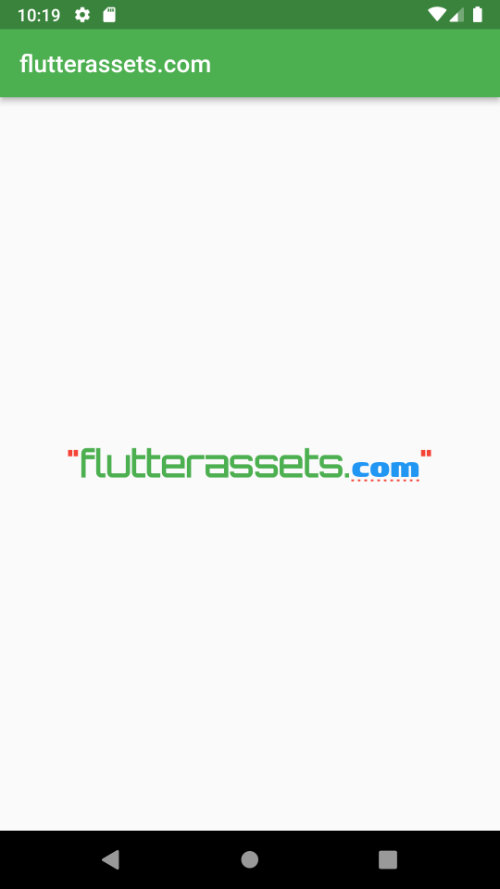
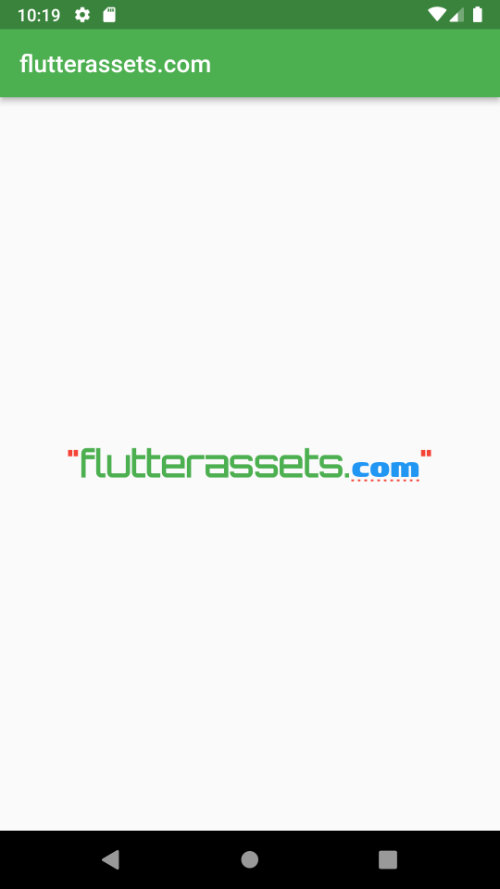
What is the main difference between RichText and Text.rich
RichText
is a widget that displays a paragraph of mixed-style text. It displays text that uses multiple different styles. Text.rich
is a constructor for the Text
widget that allows you to style a paragraph of mixed-style text.
The main difference between RichText
and Text.rich
is that RichText
is a widget and Text.rich
is a constructor. As a widget, RichText
can be inserted into the widget tree and can be styled and positioned like any other widget. Text.rich
, on the other hand, is used to construct a Text
widget and cannot be inserted into the widget tree on its own.