What can be customized in the Flutter FilterChip widget
The Flutter FilterChip
widget is similar to the ChoiceChip
widget, with some differences in how it works. You can customize several aspects of the FilterChip
widget’s appearance, such as the label text, label style, padding, shape, background colour, selected colour, disabled colour, border, elevation, and more. Additionally, FilterChip
allows for customization of the chip’s icon and the space between the icon and the label. By customizing these properties, you can create a FilterChip
that fits the look and feel of your app.
Here are the FilterChip properties:
FilterChip FilterChip({
Key? key,
Widget? avatar,
required Widget label,
TextStyle? labelStyle,
EdgeInsetsGeometry?
labelPadding,
bool selected = false,
required void Function(bool)? onSelected,
double? pressElevation,
Color? disabledColor,
Color? selectedColor,
String? tooltip,
BorderSide? side,
OutlinedBorder? shape,
Clip clipBehavior = Clip.none,
FocusNode? focusNode,
bool autofocus = false,
Color? backgroundColor,
EdgeInsetsGeometry? padding,
VisualDensity? visualDensity,
MaterialTapTargetSize? materialTapTargetSize,
double? elevation,
Color? shadowColor,
Color? surfaceTintColor,
IconThemeData? iconTheme,
Color? selectedShadowColor,
bool? showCheckmark,
Color? checkmarkColor,
ShapeBorder avatarBorder = const CircleBorder(),
})
How to use an avatar in Flutter FilterChip
To use the avatar property in the Flutter FilterChip, you can specify an avatar image that appears at the beginning of the filter chip. The avatar property takes a widget that can be used to display an image or an icon. One option for displaying an image in the avatar is to use a network image.
To use a network image as the avatar in a FilterChip, you can use the NetworkImage class to specify the image URL as a string. Then, you can pass the NetworkImage instance as the child of a CircleAvatar widget and set it as the avatar property of the FilterChip. Here’s an example code snippet:
FilterChip(
avatar: CircleAvatar(
backgroundImage: NetworkImage('https://picsum.photos/250?image=9'),
),
label: Text('Filter Chip'),
onSelected: (isSelected) {
// handle filter selection
},
)

In this example, the avatar property of the FilterChip is set to a CircleAvatar widget that has a backgroundImage property set to a NetworkImage instance. This loads the image from the specified URL and displays it as the avatar for the FilterChip.
You can customize the CircleAvatar widget to adjust the size, shape, and appearance of the avatar as desired. For example, you can use the backgroundColor property to change the background colour of the avatar or use a different radius.
Flutter FilterChip widget avatar with Image
To use an asset image in the FilterChip avatar, you can use the AssetImage class from the Flutter framework. First, you need to add your image asset to your Flutter project’s assets directory in your pubspec.yaml file. After that, you can use the AssetImage class to load the image and pass it to the FilterChip’s avatar property.
FilterChip(
label: Text('Filter Chip'),
avatar: CircleAvatar(
backgroundImage: AssetImage('assets/logo.png'),
),
onSelected: (isSelected) {},
),

Flutter FilterChip widget avatar with Icon
To use an icon in the FilterChip avatar, you can use the Icon class from the Flutter framework. You can specify the icon by passing in an IconData object that represents the icon you want to use. You can also customize the appearance of the icon using the Icon’s properties.
FilterChip(
label: Text('Filter Chip'),
avatar: CircleAvatar(
child: Icon(Icons.star),
),
onSelected: (isSelected) {},
),
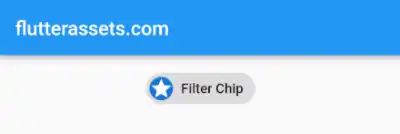
In this example, the avatar property of the FilterChip is set to a CircleAvatar widget that contains an Icon widget with the ‘star’ icon. You can customize the appearance of the icon by passing additional properties to the Icon widget, such as colour, size, and more.
How to customize the colours of the FilterChip in Flutter
In Flutter, the FilterChip widget is highly customizable when it comes to colour. Here are some of the properties that can be used to customize the FilterChip colours:
disabledColor
: This property is used to set the colour of the chip when it is disabled. It can be set to any colour value.selectedColor
: This property is used to set the background colour of the chip when it is selected. It can be set to any colour value.backgroundColor
: This property is used to set the background colour of the chip when it is not selected. It can be set to any colour value.shadowColor
: This property is used to set the colour of the shadow that appears behind the chip. It can be set to any colour value.selectedShadowColor
: This property is used to set the colour of the shadow that appears behind the chip when it is selected. It can be set to any colour value.checkmarkColor
: This property is used to set the colour of the checkmark that appears inside the chip when it is selected. It can be set to any colour value.
Here’s an example of how to use these properties to customize the FilterChip colours:
FilterChip(
label: Text('Filter Chip'),
selected: true,
onSelected: (bool selected) {},
disabledColor: Colors.grey,
selectedColor: Colors.blue,
backgroundColor: Colors.white,
shadowColor: Colors.black,
selectedShadowColor: Colors.blue,
checkmarkColor: Colors.white,
),
Each property is set to a different colour to demonstrate how they can be used to customize the appearance of the FilterChip widget.
How to use onSelected in Flutter FilterChip
The onSelected
property in the Flutter FilterChip
widget is a callback function that gets called when the filter chip gets selected. It takes a function as an argument and that function should take a boolean value as a parameter. The boolean value represents whether the filter chip is currently selected or not.
To use the onSelected
property, you can assign a function to it that you want to be called when the filter chip gets selected. For example, you could update the state of your app when the filter chip gets selected or perform some other action based on the selection status of the chip.
Here is an example code snippet that demonstrates the use of the onSelected
property in the FilterChip
widget:
bool _isSelected = false;
FilterChip(
label: Text('Filter Chip'),
selected: _isSelected,
onSelected: (isSelected) {
setState(() {
_isSelected = isSelected;
});
},
),
In this example, we first define a boolean _isSelected
variable and set it to false
. We then create a FilterChip
widget and pass in a Text
widget as the label. We set the selected
property of the FilterChip
to the _isSelected
boolean variable and we set the onSelected
property to a function that takes in the isSelected
boolean value and updates the state of the _isSelected
variable based on that value.
When the filter chip is tapped and selected, the onSelected
function is called with true
as the parameter value. The setState
function is then called to update the state of the _isSelected
variable to true
. The FilterChip
widget is then rebuilt with the new _isSelected
value, which causes it to display as selected.
How to customize the Label in the Flutter FilterChip widget
To customize the label in a Flutter FilterChip
, you can use several options such as changing the text style, using the labelPadding
property to add padding to the label, adding an icon to the label, or changing the background colour.
Changing the FilterChip label text style:
You can change the text style of the label using the TextStyle
class. For example, to change the colour of the label text to red, you can use the following code:
FilterChip(
label: Text('Filter Chip',
style: TextStyle(
color: Colors.red,
),
),
selected: true,
onSelected: (isSelected) {},
),
Changing the FilterChip label padding:
You can use the labelPadding
property to add padding to the label. For example, to add 8 pixels of padding to the label, you can use the following code:
FilterChip(
label: Text('Filter Chip'),
labelPadding: EdgeInsets.all(18.0),
selected: true,
onSelected: (isSelected) {},
),
Adding an icon to the label in FilterChip:
You can add an icon to the label by using the Row
widget and placing an Icon
widget before the Text
widget. For example, to add a checkmark icon before the label text, you can use the following code:
FilterChip(
label: Row(
children: [
Icon(Icons.star),
SizedBox(width: 8.0),
Text('Filter Chip'),
],
),
selected: true,
onSelected: (isSelected) {},
),

Changing the FilterChip label background colour:
You can change the background colour of the label by setting the backgroundColor
property. For example, to set the background colour of the label to blue, you can use the following code:
FilterChip(
label: Text('Filter Chip'),
backgroundColor: Colors.blue,
onSelected: (isSelected) {},
),
How to use selected in the Flutter FilterChip
The selected
property in Flutter FilterChip
is used to determine whether a FilterChip
is selected or not. When a FilterChip
is selected, it typically changes its colour to indicate its selection status. The selected
property is a boolean value, where true
means the FilterChip
is selected and false
means it’s not.
To use the selected
property, you need to set its value based on some logic in your app. For example, if you want to select a FilterChip
when it’s tapped, you can use the onSelected
property to update the value of selected
.
Here’s a simple code example that demonstrates the use of selected
property:
bool _isSelected = false;
FilterChip(
label: Text('Filter Chip'),
selected: _isSelected,
onSelected: (bool selected) {
setState(() {
_isSelected = selected;
});
},
),
In this example, we have created a FilterChip
with a label “Example”. The selected
property is set to _isSelected
, which is initially set to false
. The onSelected
property is used to update the _isSelected
value whenever the user taps the FilterChip
. The setState
method is used to rebuild the widget tree with the updated value of _isSelected
.
When the user taps the FilterChip
, the onSelected
callback is called with the updated value of selected
. In this example, we simply update the _isSelected
value with the new value of selected
. After that, the widget tree is rebuilt with the new value of _isSelected
. If _isSelected
is true
, the FilterChip
will be displayed with a selected colour, otherwise, it will be displayed with a default colour.
How to change the shape of the Flutter FilterChip
To change the shape of a Flutter FilterChip, you can use the shape
property. There are five types of shapes you can choose from: StadiumBorder
, RoundedRectangleBorder
, ContinuousRectangleBorder
, CircleBorder
, and BeveledRectangleBorder
.
Here’s an example code snippet that shows how to use each shape in a FilterChip:
Column(
children: [
FilterChip(
label: Text('Stadium Border'),
onSelected: (selected) {},
shape: StadiumBorder(),
),
FilterChip(
label: Text('Rounded Rectangle Border'),
onSelected: (selected) {},
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
),
FilterChip(
label: Text('Continuous Rectangle Border'),
onSelected: (selected) {},
shape: ContinuousRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
),
FilterChip(
label: Text('Continuous Rectangle Border'),
onSelected: (selected) {},
shape: ContinuousRectangleBorder(),
),
FilterChip(
label: Text('Circle Border'),
onSelected: (selected) {},
shape: CircleBorder(),
),
FilterChip(
label: Text('Circle Border'),
padding: EdgeInsets.symmetric(vertical: 40),
onSelected: (selected) {},
shape: CircleBorder(),
),
FilterChip(
label: Text('Beveled Rectangle Border'),
onSelected: (selected) {},
shape: BeveledRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
),
],
)

In this example, each FilterChip has a different shape. The StadiumBorder
creates a rounded rectangular shape with rounded ends. The RoundedRectangleBorder
creates a rectangle with rounded corners. The ContinuousRectangleBorder
creates a rectangle with square corners. The CircleBorder
creates a circular shape. Finally, the BeveledRectangleBorder
creates a rectangle with bevelled edges.
You can adjust the borderRadius
property to change the amount of rounding or beveling of the edges.
How to add a border to the Flutter FilterChip
To add a black border to a Flutter FilterChip, we can use the side
property of the ShapeBorder
class. This property allows us to specify the colour, width, and style of the border of the chip.
Here’s an example of how to add a black border to a FilterChip using the side
property:
FilterChip(
label: Text('Filter Chip Border'),
selected: true,
onSelected: (bool selected) {},
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
side: BorderSide(
color: Colors.black,
width: 2.0,
),
),
),

In this example, we’re using the RoundedRectangleBorder
shape and setting the borderRadius
property to 10.0 to create a rounded rectangle shape. Then, we’re using the side
property to add a 2-pixel-wide black border to the chip.
We can also use the other ShapeBorder
classes to create different shapes for our FilterChip, such as CircleBorder
, StadiumBorder
, BeveledRectangleBorder
, and ContinuousRectangleBorder
. To add a border to any of these shapes, we simply need to set the side
property on the shape.
For example, here’s how we could add a black border to a circular FilterChip:
FilterChip(
label: Text('Filter Chip Border'),
selected: true,
checkmarkColor: Colors.red,
padding: EdgeInsets.symmetric(vertical: 70),
onSelected: (bool selected) {},
shape: CircleBorder(
side: BorderSide(
color: Colors.black,
width: 2.0,
),
),
),
In this example, we’re using the CircleBorder
shape and setting the side
property to add a black border to the circle. We could do the same for any of the other ShapeBorder
classes, adjusting the borderRadius
or other properties as needed to achieve the desired shape.